Automatic Tracing of Java EE Applications With WildFly 14 and Jaeger
Want to learn how to enhance your Java EE application with tracing capabilities? Check out this tutorial to learn how to enable automatic tracing with Wildfly and Jaeger.
Join the DZone community and get the full member experience.
Join For FreeYou may have heard the news: WildFly 14 has shipped with an initial support for MicroProfile OpenTracing and uses Jaeger as the default tracer. This means that applications being deployed on WildFly 14 are able to get tracing capabilities just by adding one annotation on the classes and methods that need to be traced.
In this blog post, we’ll start from scratch to show you how easy it is to enhance a Java EE application with tracing capabilities.
Let’s start by downloading and starting WildFly:
curl -o wildfly-14.0.1.Final.zip http://download.jboss.org/wildfly/14.0.1.Final/wildfly-14.0.1.Final.zip
unzip -q wildfly-14.0.1.Final.zip
export WILDFLY_HOME="$(pwd)/wildfly-14.0.1.Final"
JAEGER_REPORTER_LOG_SPANS=true JAEGER_SAMPLER_TYPE=const JAEGER_SAMPLER_PARAM=1 $WILDFLY_HOME/bin/standalone.sh
The Jaeger tracer inside WildFly is configured in the same way as when used in any other service, i.e. via environment variables, with the exception of the service name. Rather than specifying the JAEGER_SERVICE_NAME environment variable, WildFly will automatically configure the service name on a per-deployment basis.
Unfortunately, WildFly 14 shipped with a bug that prevents the spans from reaching our Jaeger server. Follow the workaround described in the JIRA or download a nightly build, which should already contain the fix.
Finally, let’s get Jaeger ready as well:
docker run \
--rm \
--name jaeger \
-p6831:6831/udp \
-p16686:16686 \
jaegertracing/all-in-one:1.6
And, we kick-start our project by running gradle init
:
mkdir javaee-traced-store && cd $_
gradle init ## if you don't have Gradle yet, download it from https://gradle.org/install
Our build.gradle
is very minimal, containing only the dependency on the Java EE libraries and the MicroProfile OpenTracing library, which contains the @Traced
annotation that we need.
apply plugin: 'war'
group = 'io.jaegertracing.examples.wildfly'
version = '1.0-SNAPSHOT'
description = "JavaEE Traced Store"
sourceCompatibility = 1.8
targetCompatibility = 1.8
repositories {
mavenLocal()
mavenCentral()
}
dependencies {
providedCompile 'javax:javaee-api:8.0'
providedCompile 'org.eclipse.microprofile.opentracing:microprofile-opentracing-api:1.1'
}
At this point, we should be able to build a Java Web Archive (WAR) for our project: run ./gradlew war
and check your build/lib
directory.
Right now, our application isn’t doing anything. Let’s add a simple JAX-RS marker class so that we are able to create REST endpoints:
// src/main/java/io/jaegertracing/examples/wildfly/TracedStoreApplication.java
package io.jaegertracing.examples.wildfly;
import javax.ws.rs.ApplicationPath;
import javax.ws.rs.core.Application;
@ApplicationPath("/")
public class TracedStoreApplication extends Application {
}
The next step is to create our endpoint. Note how we add the @Stateless
annotation to the JAX-RS endpoint so that we get EJB features as well. It is common practice to do this in the real world, but at this point for us, the only effect is getting our deployment marked as “CDI archive,” which is enough to trigger the MicroProfile OpenTracing integration.
// src/main/java/io/jaegertracing/examples/wildfly/OrderEndpoint.java
package io.jaegertracing.examples.wildfly;
import javax.ejb.Stateless;
import javax.ws.rs.POST;
import javax.ws.rs.Path;
@Stateless
@Path("/order")
public class OrderEndpoint {
@POST
@Path("/")
public String placeOrder() {
return "Order placed";
}
}
Once we build and the deployment completes, we can call this endpoint via curl
:
./gradlew war
cp build/libs/javaee-traced-store-1.0-SNAPSHOT.war $WILDFLY_HOME/standalone/deployments/
# watch the WildFly logs and wait for the deployment to finish
curl -X POST localhost:8080/javaee-traced-store-1.0-SNAPSHOT/order
You should be able to see one trace in the Jaeger UI for the service javaee-traced-store-1.0-SNAPSHOT.war
, containing one span. We can make our store fancier by adding a new bean, AccountService
:
// src/main/java/io/jaegertracing/examples/wildfly/AccountService.java
package io.jaegertracing.examples.wildfly;
import javax.ejb.Stateless;
import org.eclipse.microprofile.opentracing.Traced;
@Stateless
@Traced
public class AccountService {
public void onNewOrder() {
// we would do something interesting here
}
}
Our new class is both an EJB and a CDI bean and can be injected as such into our JAX-RS endpoint. Let’s inject it there and call the new method from our bean:
// src/main/java/io/jaegertracing/examples/wildfly/OrderEndpoint.java
package io.jaegertracing.examples.wildfly;
import javax.inject.Inject;
import javax.ws.rs.POST;
import javax.ws.rs.Path;
@Path("/order")
public class OrderEndpoint {
@Inject
AccountService accountService;
@POST
@Path("/")
public String placeOrder() {
accountService.onNewOrder();
return "Order placed";
}
}
To see what it looks like now in a trace, let’s build, deploy, and run it once more:
./gradlew war
cp build/libs/javaee-traced-store-1.0-SNAPSHOT.war $WILDFLY_HOME/standalone/deployments/
# watch the WildFly logs and wait for the deployment to finish
curl -X POST localhost:8080/javaee-traced-store-1.0-SNAPSHOT/order
At this point, we should now see a second trace for our service, but with two spans.
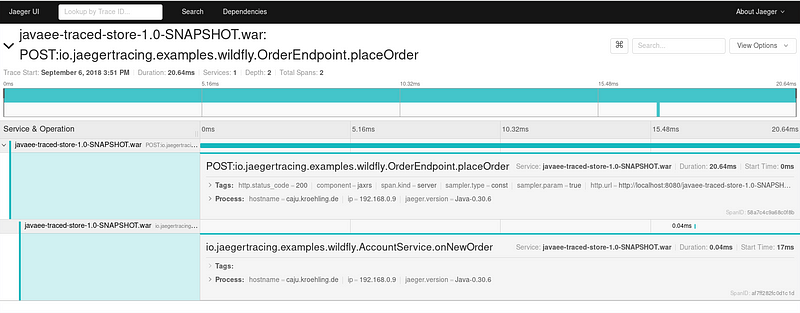
Our example is purposefully simple: to demonstrate that a single annotation is all it takes to get tracing information for our Java EE application deployed on WildFly 14. Try this new integration out with your existing applications and share your feedback in the comments below!
Published at DZone with permission of Juraci Paixao Kroehling, DZone MVB. See the original article here.
Opinions expressed by DZone contributors are their own.
Comments