All Things Java 8 [Tutorials]
No matter what version of the JDK we are on, Java 8 is not going anywhere.
Join the DZone community and get the full member experience.
Join For Free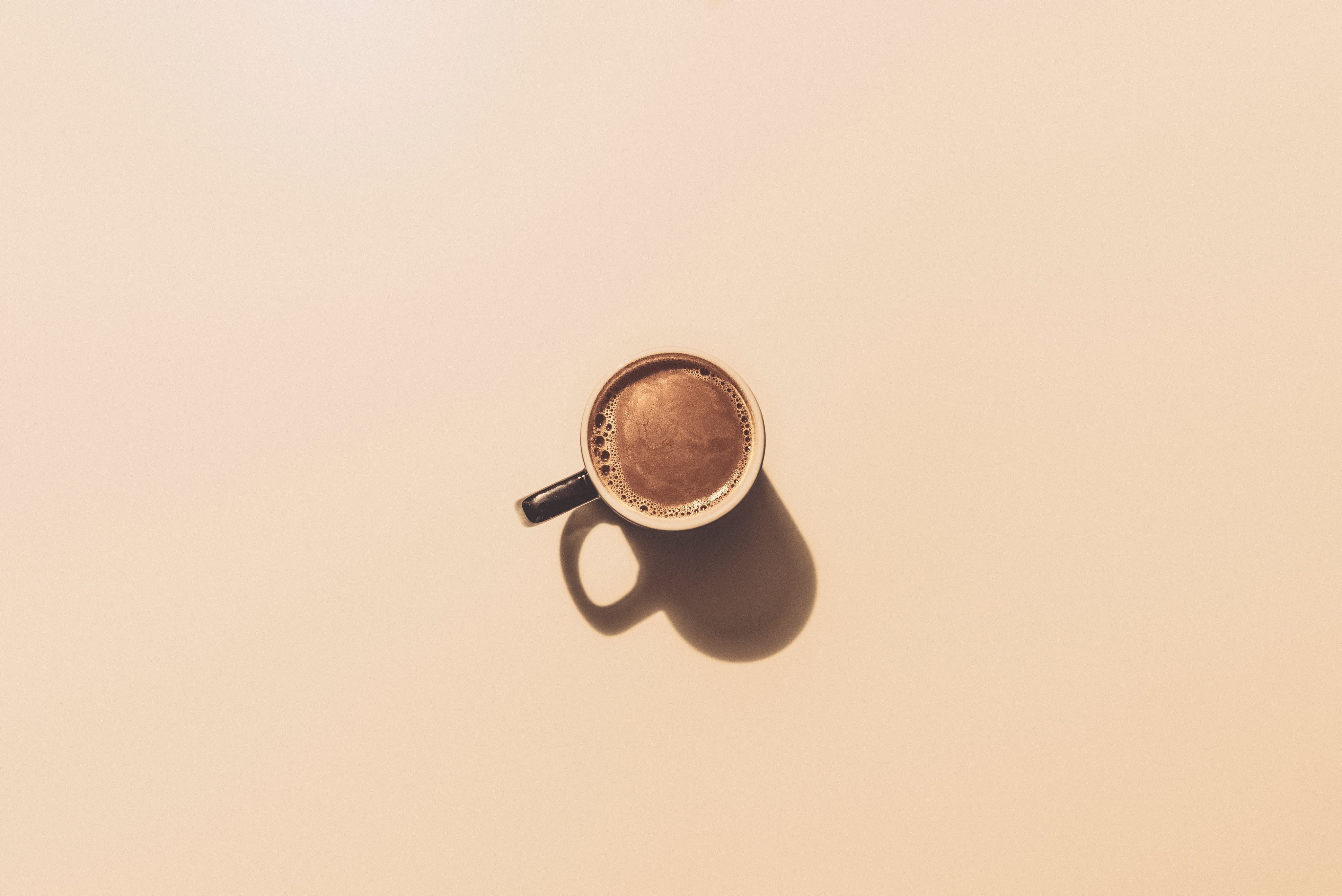
Java 8 introduced a new era of Java. Everything from lambda expressions and functional programming to Streams and collections — DZone was there to document it all.
So whether you're migrating over to Java 9 or Java 11, or maybe even Java 13, Java 8 concepts and features are still very much alive in the JDK. And understanding these core concepts can help tremendously when it's time to move beyond Java 8.
So without further ado, here are the top DZone articles and tutorials on all things Java 8. Enjoy!
The Basics
Java 8 (A Comprehensive Look) — This article is a great starting point when looking at Java 8, with a special focus on functional programming.
Java 8 Concepts: FP, Lambda Expressions, and Streams — This technical overview of Java 8 covers major concepts, including translations to the imperative paradigms, and how streams and lambdas work.
Java 8 Comparator: How to Sort a List — This post help to sort a list in Java 8 using the
Comparator
method, demonstrating how to sort a list of strings by various classifications.A Guide to Java's SimpleDateFormat — Java's
SimpleDateFormat
class comes with a variety of choices for formatting dates and times. This article covers all of your options, but beware, it's not thread-safe.Java 8: The Bad Parts — Explore Java 8's Stream API, lambda expressions, and
CompletableFuture
to see what functionality they brought, but also how they could be improved.When Will Java 11 Replace Java 8 as the Default Java? — tl;dr Java 8 isn't going anywhere.
What Future Java Releases Will Mean for Legacy Desktop Apps — Java 11 will mean the end of Java Web Start. Let's see what this means for legacy desktop apps and how developers can be ready for the change.
Java 8 Optional: Handling Nulls Properly — This tutorial teaches you how to handle null properties in Java 8 by using the
Optional
class to help reduce the number of errors in nulls.Using Java Enums — Check out this post to learn more about ideal use cases and best practices for using enums in Java, including how to maintain type safety using enums.
Random Number Generation in Java — Need some randomness in your numbers? Check out a few different techniques you can avail yourself of to create random (or mostly random) numbers in Java.
The Push Towards Functional Programming
Functional Programming With Java 8 Functions — Check out this post to learn how to use lambda expressions and anonymous functions in Java 8.
Using Java 8? Please Avoid Functional Vomit — More characters do not equal worse code. This post — particularly the comments section — has some interesting thoughts on writing clean Java code.
Check out this series, Functional Programming in Java 8, by Niklas Wuensche, covering Java 8 topics such as Motivation, Functions as Objects, Optionals, Streams, and Splitter.
Functional Programming Patterns With Java 8 — This tutorial provides exercises from traditional, imperative-style code to functional-style code in Java 8, continuously aiming to write cleaner code.
Java 8 Lambda Expressions
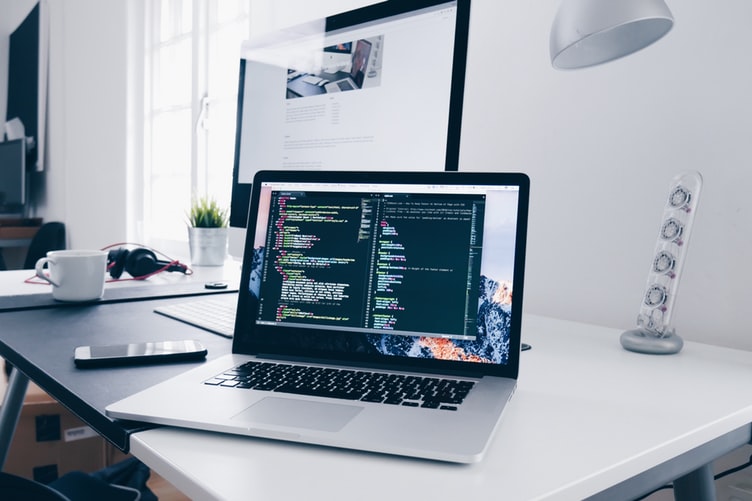
Lambda Expressions in Java 8 — In this post, we learn what, exactly, lambda expressions are and how they fit into the whole Java ecosystem.
More on Lambda Expressions in Java 8 —If you're ever having trouble grasping Java 8's lambda expressions, you aren't alone. This article will help you see how to get your lambda expressions up and running.
How to Handle Check Exceptions With Lambda Expression — This tutorial demonstrates how devs can handle checked exceptions in their projects using Java 8 and lambda expressions for cleaner, more concise code.
Java Lambda: Method References — In this post, we take a look at a crucial part of Java lambdas, the method reference, specifically looking at the static reference, instance methods, and more.
Functional Interface and Lambda Expressions in Java 8 — This post takes a look at using abstract methods in Java 8 with the functional interface and lambda expressions, specifically methods with different inputs.
How to Use Java HashMap Effectively — This post explores the best practices for implementing
HashMap
s in Java 8 and onwards, identifying values or keys, lambda expressions, and more.Don't Fear the Lambda — This post teaches you when to use lambda expressions in Java and how to use them to refactor your code into neater, more readable snippets, accompanied with some examples, of course.
Java Interfaces & Default Methods
Java 8 History: Interfaces — Check out this post to see what Java 8 brought to Interfaces, including default methods, rules for multiple inheritance, shared methods, and static methods.
Interface Default Methods in Java 8 — This tutorial looks at how to use interface default methods in Java 8, which allows devs to add methods to the interfaces without breaking existing ones.
Cheatsheet: Java Functional Interfaces — This compilation of the 43 functional interfaces in Java covers their intended uses and tips for implementing them properly.
When to Use Java 8 Default Methods in Interfaces — This article looks at the Oracle documentation on when default methods should be used and breaks down what this means for interfaces.
Default and Private Methods in Interfaces — Java 8 brought default methods to interfaces, and Java 9 promises to bring in private methods. This post looks at both in action and how to keep your code clean.
Java 8 Streams & Collections
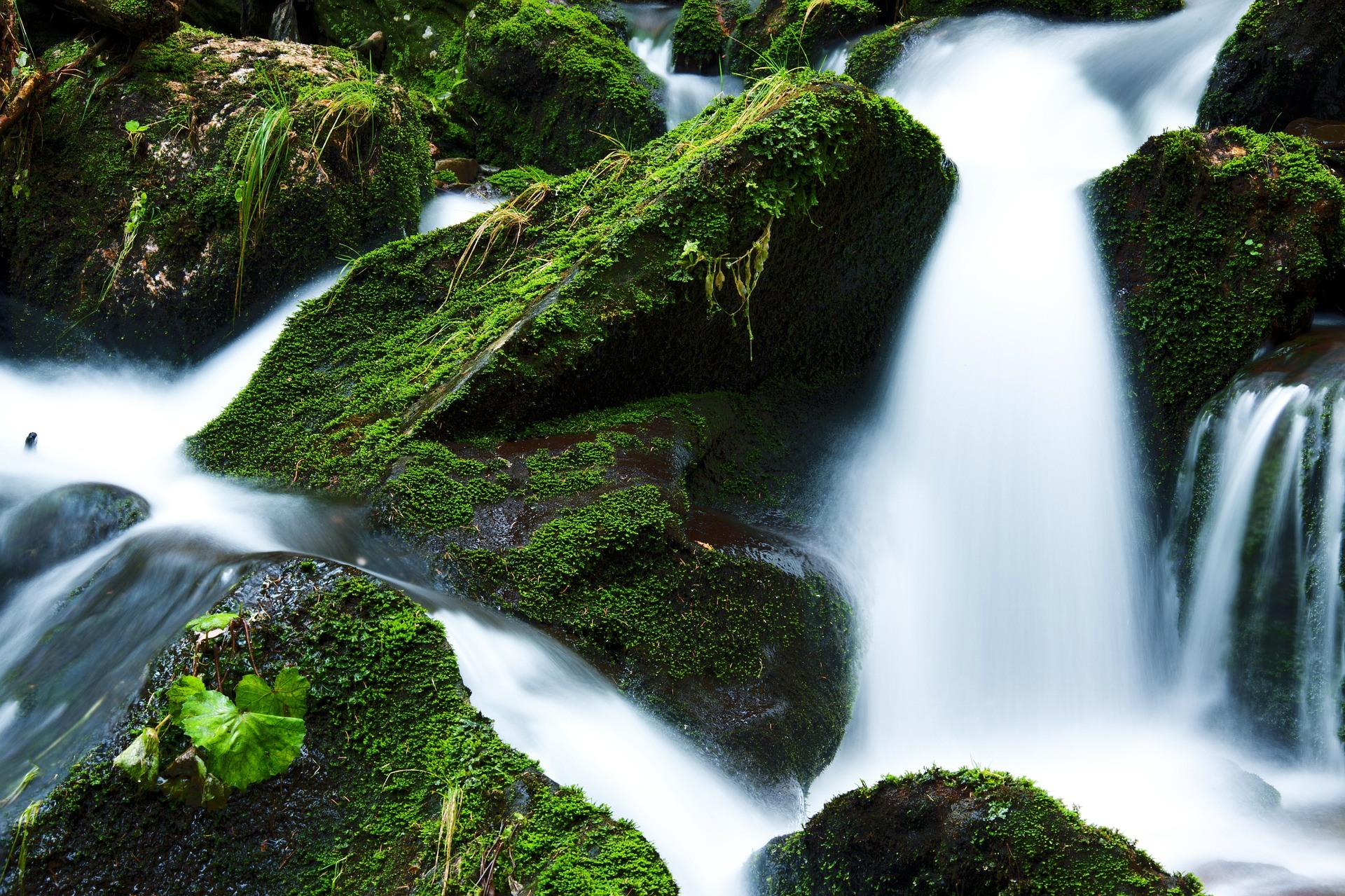
The Developer's Guide to Collections — This series by Justin Albano covers the collection interface, associated methods to help with collections, and general implementation advice.
Process Collections Easily With Stream in Java 8 — This tutorial teaches us how to use Streams to easily process collections in Java 8, looking specifically at characteristics, advantages, and disadvantages.
Java 8 Map, Filter, and Collect Examples — Learn how to use the
map()
function in Java 8 to transform an object to another and how to use thefilter()
to select an object-based upon condition.Java 8 Streaming Example With CSV File — This example showcases the day-to-day use cases of an aggregate function through the Java 8 Streaming API.
Understanding FlatMap With Java Streams — This article introduces a crucial concept for functional programming in Java — see how
flatMap
s work step by step, then examines what they bring to Streams and Optionals.Iteration Over Java Collections With High Performance — This post looks at collections in Java, specifically the
forEach
loop and how it compares to C-style and Stream API, concluding that rewriting loops is most effective.A Guide to Java 8 Streams: In-Depth Tutorial With Examples — Java 8's introduction of Streams can be overwhelming. This post dives into Streams and sees how best to use them in your code.
The Java 8 API
API Design With Java 8 — Learn to be a better Java programmer by mastering Java 8 API design, exposing a well-designed API, making sure that client code can use lambdas, and more.
Java 8 Date and Time API — This post journies back to the not-too-distant past to see how Java 8 improved date and time APIs and how to put them to use.
API Enhancements Missing Since Java 8 — This post presents some API enhancements that could make Java API more user-friendly, without breaking backward compatibility. Readers shared mixed views. Give it a read and let us know your thoughts.
20 Examples of Using Java's CompletableFuture —For a better understanding of the
CompletionStage
API, let's look at 20 examples ofCompletableFuture
in action, both synchronously and asynchronously.Interface Enhancement in Java 8 — Interfaces have seen massive improvements since they were first introduced. Combined with method definitions, you've got a lot of flexibility on your hands.
Java String (With Examples)
Java String Formatting Examples — This tutorial offers a brief overview, several examples, and a collection of references to keep by your side whenever you are formatting Java strings.
Concatenating Strings in Java 8 — Brush up on your string concatenation skills with this look at what Java 8 brought, including working with Streams and Optionals, as well as general advice.
We Don't Need StringBuilder for Simple Concatenation — Concatenating strings is useful, but expensive. Fortunately, you don't need to use
StringBuilder
anymore — the compiler can handle it for you.Think Twice Before Using Java 8 Parallel Streams — Parallelization was the main driving force behind lambdas, stream API, and others. This post looks at why you should think twice before using this feature.
Convert a List to a Comma-Separated String in Java 8 — This tutorial demonstrates how to use streams in Java 8 and Java 7 to convert a list to a comma-separated string by manipulating the string before joining.
Opinions expressed by DZone contributors are their own.
Comments