Understanding Loose Typing in JavaScript
Join the DZone community and get the full member experience.
Join For Freefor many front end developers, javascript was their first taste of a scripting and/or interpretive language. to these developers, the concept and implications of loosely typed variables may be second nature. however, the explosive growth in the demand for web 2.0-ish applications has resulted in a growing number of back end developers that have had to dip their feet into pool of client side technologies. many of these developers are coming from a background in strongly typed languages, such as c# and java, and are unfamiliar with both the freedom and the potential pitfalls involved in working with loosely typed variables.
since the concept of loose typing is so fundamental to scripting in javascript, an understanding of it is essential. this article is a top level discussion of loose typing in javascript. since there may be subtle differences in loose typing from language to language, let me constrain this discussion to the context of javascript. ok, let's dig in...
what is loose typing?
well, this seems like a good place to start. it is important to understand both what loose typing is , and what loose typing is not . loose typing means that variables are declared without a type. this is in contrast to strongly typed languages that require typed declarations. consider the following examples:
/* javascript example (loose typing) */
var a = 13; // number declaration
var b = "thirteen"; // string declaration
/* java example (strong typing) */
int a = 13; // int declaration
string b = "thirteen"; // string declaration
notice that in the javascript example, both a and b are declared as type var. please note, however, that this does not mean that they do not have a type, or even that they are of type "var". variables in javascript are typed, but that type is determined internally. in the above example, var a will be type number and var b will be type string. these are two out of the three primitives in javascript, the third being boolean.
javascript also has other types beyond primitives. the type diagram for javascript is as follows (as per mozilla ):
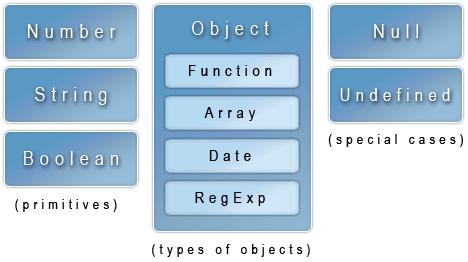
ya really - null and undefined too.
note, however, that this distinction between primitives and objects will be dismissed in javascript 2.0. you can read more about that here .
type coercion
type coercion is a topic that is closely associated with loose typing. since data types are managed internally, types are often converted internally as well. understanding the rules of type coercion is extremely important. consider the following expressions, and make sure you understand them:
7 + 7 + 7; // = 21
7 + 7 + "7"; // = 147
"7" + 7 + 7; // = 777
in the examples above, arithmetic is carried out as normal (left to right) until a string is encountered. from that point forward, all entities are converted to a string and then concatenated.
type coercion also occurs when doing comparisons. you can, however, forbid type coercion by using the === operator. consider these examples:
1 == true; // = true
1 === true; // = false
7 == "7"; // = true
7 === "7"; // = false;
there are methods to explicitly convert a variable's type as well, such as parseint and parsefloat (both of which convert a string to a number).
double negation (!!) can also be used to cast a number or string to a boolean. consider the following example:
true == !"0"; // = false
true == !!"0"; // = true
conclusion
this obviously is not a definitive reference to loose typing in javascript (or type coercion for that matter). i do hope, however, that this will be a useful resource to those who are not familiar with these topics, and a good refresher for those who already are. i have tried to insure that the above is accurate, but if you notice anything incorrect, please let me know! and as always, thanks for reading!
Published at DZone with permission of Jeremy Martin. See the original article here.
Opinions expressed by DZone contributors are their own.
Comments