Starting Off With LogT
In this article, we will be talking about LogT and how to use it. This particular library is distinguished by its logs and by the importance of making its labels unique.
Join the DZone community and get the full member experience.
Join For FreeIn researching loggers, I noticed this library LogT. This frontend tool is different from other logs with its unique feature of colorful labels.
The term log refers to a logarithmic function or a log statement in a program. In coding, a log statement is used to record events or messages during the execution of a program, usually for debugging.
LogT is a colorful logger made for browsers. You can use this logger for your front-end projects.
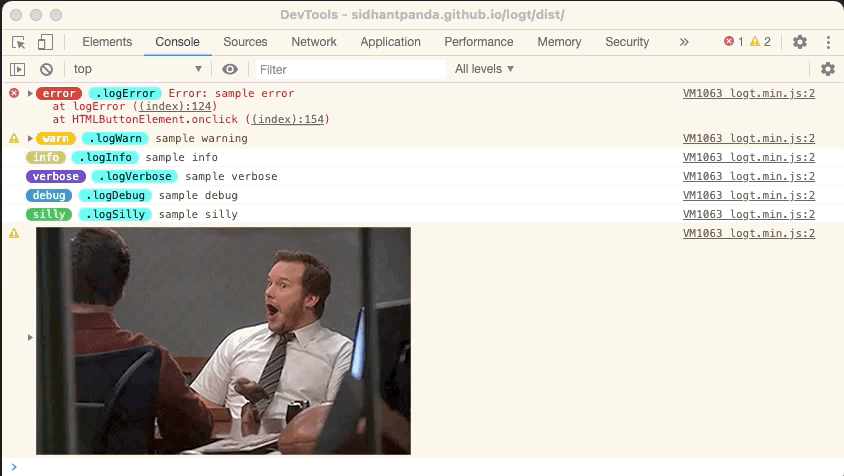
Features of LogT
- Colorful labels: This particular library is distinguished by its logs and by the importance of making its labels unique.
- Library size: This library occupies only 1.46KB of gzip, which means that it is tiny.
- Only logs equal to or above logLevel will be shown, showing log messages that are not visible to the logger.
The logs that the log level shows are stated below:
const logger = new LogT(0);
logger.warn("TAG", "warning message"); // Will not print anything to console
logger.info("TAG", "info message"); // Will not print anything to console
logger.debug("TAG", "debug message"); // Will not print anything to console
logger.silly("TAG", "silly message"); // Will not print anything to console
logger.showHidden(1); // Will print the warning message
logger.showHidden(2); // Will print the info message
logger.showHidden(5); // Will print the debug as well as silly message
As we can see in the code block above, the words that the logger will print to the console and the ones it won't print.
- Hides less important log messages with its log level
- To use the custom logger, you have to override the default console methods anywhere on the web page. This is where the read console method comes in
readConsole()
.
In replacing the default console.error,
console.warm
, console.log
, and console.info
, implement the logtlogger
.
Example:
const logger = new LogT(0);
logger.readConsole();
console.error(new Error("test error")); // will be same as logger.error('console', new Error('test error'));
console.warn("warn message"); // will be same as logger.warn('console', 'warn message');
console.log("info message"); // will be same as logger.info('console', 'info message');
console.log("log message"); // will be same as logger.debug('console', 'log message');
With the code blocks above, we can see how the custom logger logToverrides
the default console.
- Its detailed type information is built with typescript and its autocomplete format.
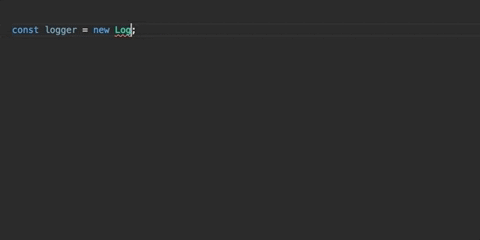
Installation
To install this library, run the following command below:
$ npm i logt -S
When you are done installing, go to your package.jason. This is how it would look, according to the version:
"logt": "^1.4.5",
Usage
This logger can be used for front-end projects, either as an ES6 module or directly in HTML.
As an ES6 Module
ES6 is a feature of the ECMAScript 6 (ES6) language specification that provides a way to organize and share Javascript code in a modular way.
Create a file and name it logger.ts
.
import LogT from "logt";
const LOG_TAG = "sample tag";
let logger;
if (process.env.NODE_ENV === "production") {
logger = new LogT("error"); // or logger = new LogT("none");
} else {
logger = new LogT("silly");
}
//To see the documentation for readConsole() for usage.
// logger.readConsole();
logger.error(LOG_TAG, new Error("example error"));
Usage in HTML
LogT is also used in HTML; you can see it in the code blocks below:
<script src="https://cdn.jsdelivr.net/gh/sidhantpanda/logt/dist/logt.min.js"></script>
<script>
var LOG_TAG = 'sample tag';
var logger = createLogger('error');
//To see the documentation for readConsole() for usage.
// logger.readConsole();
logger.error(LOG_TAG, new Error('example error'));
</script>
Documentation
Logger initialization: In computer programming, logger initialization refers to the process of setting up and configuring a logging module in your software application. The purpose of a logger is to log messages from the application, such as errors, warnings, and informational messages, which can be used for debugging, performance analysis, and auditing purposes. During the logger initialization process, you typically specify the logging level, output destination (such as a file, the console, or a remote server), and the format of the log messages.
The logger initialization in logT is stated in the code block below:
import LogT from "logt";
let logger;
// Available log levels - -1 | 0 | 1 | 2 | 3 | 4 | 5 | 'none' | 'error' | 'warn' | 'info' | 'verbose' | 'debug' | 'silly';
// noneLogger will print nothing
noneLogger = new LogT(-1); // or
noneLogger = new LogT("none");
// if included via HTML script
noneLogger = createLogger(-1); // or
noneLogger = createLogger("none");
// errorLogger will only error messages
errorLogger = new LogT(0); // or
errorLogger = new LogT("error");
// if included via HTML script
errorLogger = createLogger(0); // or
errorLogger = createLogger("error");
// sillyLogger will print all messages
sillyLogger = new LogT(5); // or
sillyLogger = new LogT("silly");
// if included via HTML script
sillyLogger = createLogger(5); // or
sillyLogger = createLogger("silly");
If any other value is supplied to the constructor, a default value of none is used.
Conclusion
In creating or building your colorful logger, this is the best library to use for your framework.
Opinions expressed by DZone contributors are their own.
Comments