Sprint Boot, REST, MongoDB POC
In this article, I’m going to cover Spring boot, Rest, and Mongo DB applications.
Join the DZone community and get the full member experience.
Join For FreeSpring Boot + Rest + Mongo DB POC
Spring Boot
Spring boot is one of the hot technologies in the market that provides all on-demand libraries to make easy of developer work. When I look back journey of Sprint from 3.x to Spring boot 2.x, there are a lot of changes and flexibilities provided by the framework. Spring boot bootstraps the application development process. In this article, I’m going to cover Spring boot, Rest, and Mongo DB application.
Mongo DB
Mongo DB is a document database, you can create data in different formats and persist JSON directly into Mongo DB.
Scope
Develop rest APIs for Quotes services which should provide all CURD operations on QuoteServiceAPI. I intend to keep it as simple as possible to make Everyone understands easily. Once you understand the blow then you can make however you want.
Technology Stack: Spring Boot 2.2.0, Mongo DB, Java 8 and Spring Rest
CodeBase
Go to start.spring.io/ and initialize the starter dependencies mentioned below.
POM.XML
Code Structure
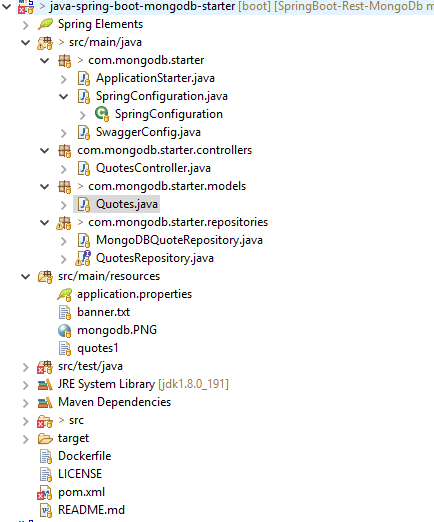
Repository:
QuotesRepository.java (I)
package com.mongodb.starter.repositories;
import static com.mongodb.client.model.Filters.eq;
import java.util.ArrayList;
import java.util.List;
import org.bson.types.ObjectId;
import org.springframework.stereotype.Repository;
import com.mongodb.starter.models.Quotes;
/*
* author : Rajesh Thokala
* date : 29/05/2020
*/
public interface QuotesRepository {
Quotes save(Quotes quotes);
List<Quotes> saveAll(List<Quotes> quotes);
List<Quotes> findAll();
List<Quotes> findAll(List<String> ids);
Quotes findOne(String id);
long count();
long delete(String id);
long delete(List<String> ids);
long deleteAll();
Quotes update(Quotes quotes);
long update(List<Quotes> quotess);
List<Quotes> finAllByType(String type);
/*added to find by type **/
List<Quotes> finByType(String type);
public List<Quotes> finAllByAuthor(String author);
/*added to find by type **/
List<Quotes> finByAuthor(String author);
}
MongoDBQuoteRepository.java©
package com.mongodb.starter.repositories;
import static com.mongodb.client.model.Filters.eq;
import static com.mongodb.client.model.Filters.in;
import static com.mongodb.client.model.ReturnDocument.AFTER;
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
import javax.annotation.PostConstruct;
import org.bson.BsonDocument;
import org.bson.types.ObjectId;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Repository;
import com.mongodb.ReadConcern;
import com.mongodb.ReadPreference;
import com.mongodb.TransactionOptions;
import com.mongodb.WriteConcern;
import com.mongodb.client.ClientSession;
import com.mongodb.client.MongoClient;
import com.mongodb.client.MongoCollection;
import com.mongodb.client.model.FindOneAndReplaceOptions;
import com.mongodb.client.model.ReplaceOneModel;
import com.mongodb.client.model.WriteModel;
import com.mongodb.starter.models.Quotes;
/*
* author : Rajesh Thokala
* date : 29/05/2020
*/
public class MongoDBQuoteRepository implements QuotesRepository {
private static final TransactionOptions txnOptions = TransactionOptions.builder()
.readPreference(ReadPreference.primary())
.readConcern(ReadConcern.MAJORITY)
.writeConcern(WriteConcern.MAJORITY)
.build();
private MongoClient client;
private MongoCollection<Quotes> quotesCollection;
int count=0;
void init() {
quotesCollection = client.getDatabase("test").getCollection("quotes", Quotes.class);
}
public Quotes save(Quotes quote) {
quote.setId(new ObjectId());
quotesCollection.insertOne(quote);
return quote;
}
public List<Quotes> saveAll(List<Quotes> quotes) {
try (ClientSession clientSession = client.startSession()) {
return clientSession.withTransaction(() -> {
quotes.forEach(p -> p.setId(new ObjectId()));
quotesCollection.insertMany(clientSession, quotes);
return quotes;
}, txnOptions);
}
}
/*added to find all by type **/
public List<Quotes> finAllByAuthor(String author){
return quotesCollection.find(eq("author",author)).into(new ArrayList<>());
// return quotesCollection.find(eq("author",author)).batchSize(3).into(new ArrayList<>());
}
/*added to find by type **/
public List<Quotes> finByAuthor(String author){
List<Quotes> quoteList= quotesCollection.find(eq("author",author)).into(new ArrayList<>());
List<Quotes> newList=new ArrayList<>();
for(int i=count;i<count+3&&i<quoteList.size();i++) {
newList.add(quoteList.get(i));
}
return newList;
}
/*added to find all by type **/
public List<Quotes> finAllByType(String type){
return quotesCollection.find(eq("type",type)).into(new ArrayList<>());
}
/*added to find by type **/
public List<Quotes> finByType(String type){
List<Quotes> quoteList= quotesCollection.find(eq("type",type)).into(new ArrayList<>());
List<Quotes> newList=new ArrayList<>();
for(int i=count;i<count+3&&i<quoteList.size();i++) {
newList.add(quoteList.get(i));
}
return newList;
}
public List<Quotes> findAll() {
// TODO Auto-generated method stub
return quotesCollection.find().into(new ArrayList<>());
}
public List<Quotes> findAll(List<String> ids) {
// TODO Auto-generated method stub
return quotesCollection.find(in("_id", mapToObjectIds(ids))).into(new ArrayList<>());
}
public Quotes findOne(String id) {
// TODO Auto-generated method stub
return quotesCollection.find(eq("_id", new ObjectId(id))).first();
}
public long count() {
// TODO Auto-generated method stub
return quotesCollection.countDocuments();
}
public long delete(String id) {
// TODO Auto-generated method stub
return quotesCollection.deleteOne(eq("_id", new ObjectId(id))).getDeletedCount();
}
public long delete(List<String> ids) {
// TODO Auto-generated method stub
try (ClientSession clientSession = client.startSession()) {
return clientSession.withTransaction(
() -> quotesCollection.deleteMany(clientSession, in("_id", mapToObjectIds(ids))).getDeletedCount(),
txnOptions);
}
}
public long deleteAll() {
// TODO Auto-generated method stub
try (ClientSession clientSession = client.startSession()) {
return clientSession.withTransaction(
() -> quotesCollection.deleteMany(clientSession, new BsonDocument()).getDeletedCount(), txnOptions);
}
}
public Quotes update(Quotes quotes) {
// TODO Auto-generated method stub
FindOneAndReplaceOptions options = new FindOneAndReplaceOptions().returnDocument(AFTER);
return quotesCollection.findOneAndReplace(eq("_id", quotes.getId()), quotes, options);
}
public long update(List<Quotes> quotes) {
// TODO Auto-generated method stub
List<WriteModel<Quotes>> writes = quotes.stream()
.map(p -> new ReplaceOneModel<>(eq("_id", p.getId()), p))
.collect(Collectors.toList());
try (ClientSession clientSession = client.startSession()) {
return clientSession.withTransaction(
() -> quotesCollection.bulkWrite(clientSession, writes).getModifiedCount(), txnOptions);
}
}
private List<ObjectId> mapToObjectIds(List<String> ids) {
return ids.stream().map(ObjectId::new).collect(Collectors.toList());
}
}
Controller:
QuotesController.Java
package com.mongodb.starter.controllers;
import static java.util.Arrays.asList;
import java.util.List;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.DeleteMapping;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.PutMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseStatus;
import org.springframework.web.bind.annotation.RestController;
import com.mongodb.starter.models.Quotes;
import com.mongodb.starter.repositories.QuotesRepository;
/*
* author : Rajesh Thokala
* date : 29/05/2020
*/
"/api") (
public class QuotesController {
private final static Logger LOGGER = LoggerFactory.getLogger(QuotesController.class);
private final QuotesRepository quotesRepository;
public QuotesController(QuotesRepository quotesRepository) {
this.quotesRepository = quotesRepository;
}
"quote") (
HttpStatus.CREATED) (
public Quotes postQuotes( Quotes quote) {
return quotesRepository.save(quote);
}
"quotes") (
HttpStatus.CREATED) (
public List<Quotes> postQuotess( List<Quotes> Quotes) {
return quotesRepository.saveAll(Quotes);
}
"quotes") (
public List<Quotes> getQuotess() {
return quotesRepository.findAll();
}
"quote/{id}") (
public ResponseEntity<Quotes> getQuotes( String id) {
Quotes quotes = quotesRepository.findOne(id);
if (quotes == null)
return ResponseEntity.status(HttpStatus.NOT_FOUND).build();
return ResponseEntity.ok(quotes);
}
/** Getting list of quotes with type ***/
"quotes/type/{all}") (
public List<Quotes> getQuotesByType( String all){
return quotesRepository.finAllByType(all);
}
/** Getting quote with type ***/
"quote/type/{findOne}") (
public List<Quotes> getQuoteByType( String findOne){
return quotesRepository.finByType(findOne);
}
/** Getting list of quotes with author ***/
"quotes/author/{all}") (
public List<Quotes> getQuotesByAuthor( String all){
return quotesRepository.finAllByAuthor(all);
}
/** Getting quote with author ***/
"quote/author/{findOne}") (
public List<Quotes> getQuoteByAuthor( String findOne){
return quotesRepository.finByAuthor(findOne);
}
"quotes/{ids}") (
public List<Quotes> getQuotess( String ids) {
List<String> listIds = asList(ids.split(","));
return quotesRepository.findAll(listIds);
}
"quotes/count") (
public Long getCount() {
return quotesRepository.count();
}
"quotes/{id}") (
public Long deleteQuotes( String id) {
return quotesRepository.delete(id);
}
"quotes/{ids}") (
public Long deleteQuotess( String ids) {
List<String> listIds = asList(ids.split(","));
return quotesRepository.delete(listIds);
}
"quotes") (
public Long deleteQuotess() {
return quotesRepository.deleteAll();
}
"quote") (
public Quotes putQuote( Quotes quote) {
return quotesRepository.update(quote);
}
"quotes") (
public Long putQuotes( List<Quotes> quotes) {
return quotesRepository.update(quotes);
}
RuntimeException.class) (
HttpStatus.INTERNAL_SERVER_ERROR) (
public final Exception handleAllExceptions(RuntimeException e) {
LOGGER.error("Internal server error.", e);
return e;
}
}
MongoDB
Get complete code from below GIT link https://github.com/rajeshjaava/SpringBoot-Rest-MongoDb.
Thanks for reading the article!
Opinions expressed by DZone contributors are their own.
Comments