Introduction To Face Authentication With FACEIO in AngularJS
This is a step-by-step guide illustrating how to implement face authentication via FaceIO in Angular JS.
Join the DZone community and get the full member experience.
Join For FreeIn today’s digital age, security, and user convenience are of paramount importance for web applications. Traditional methods of authentication, such as passwords, while widely used, come with their own set of challenges, including the risk of breaches and the inconvenience of remembering and managing passwords. In this context, facial authentication offers an innovative and secure solution that enhances the user experience.
This guide introduces you to the world of face authentication using the FACEIO platform, specifically tailored for AngularJS applications. FACEIO simplifies the integration of facial recognition technology, allowing you to incorporate this advanced security feature seamlessly into your web applications.
The Power of Facial Authentication
Face authentication, as the name suggests, is a biometric authentication method that uses an individual’s facial features for identity verification. It leverages the uniqueness of a person’s face, making it incredibly difficult for unauthorized users to gain access. Unlike traditional passwords or PINs, a face is something a user always carries with them.
The benefits of facial authentication are numerous:
- Enhanced Security: Facial authentication provides a high level of security. A user’s face is unique, and the technology can detect even subtle differences, making it extremely difficult to spoof.
- User Convenience: Users appreciate the simplicity of facial authentication. There’s no need to remember complex passwords or PINs, reducing the risk of forgotten credentials.
- Fast and Frictionless: The process of face authentication is swift and non-intrusive. Users simply look into the camera, and access is granted almost instantly.
- Contactless: In an era where health and safety are paramount, facial authentication is entirely contactless, reducing the risk of disease transmission.
Why AngularJS and FACEIO?
AngularJS, a popular JavaScript framework, is widely used for building dynamic web applications. Combining AngularJS with FACEIO allows you to create secure, user-friendly, and efficient applications that leverage the power of facial authentication.
In this guide, we’ll walk you through the step-by-step process of integrating FACEIO into your AngularJS application. You’ll learn how to set up FACEIO, enroll users, and configure security settings. You’ll also discover how to implement webhooks for real-time event notifications and handle webhooks in your application.
By the end of this guide, you’ll be equipped to enhance the security of your AngularJS applications and provide your users with a seamless, contactless, and highly secure authentication experience using facial recognition technology. It’s time to embrace the future of authentication with FACEIO and AngularJS. Let’s get started!
Step 1: Set Up an AngularJS Project
Install Node.js and npm (Node Package Manager)
Visit the official Node.js website and download the latest version.
Follow the installation instructions provided for your operating system.
Install AngularJS Globally:
Open your preferred Command Line Interface (CLI).
Run the following command to install AngularJS globally:
npm install -g @angular/cli
Create a New AngularJS Project
Use the AngularJS CLI to create a new project. Run this command:
ng new my-angular-project
Replace my-angular-project with your desired project name.
Navigate To Your Project Directory
Move to the newly created project directory using this command:
cd my-angular-project
Serve Your AngularJS Application
Start the development server and open your project in a web browser:
ng serve
Step 2: Create a FACEIO Account
Visit the FACEIO Console:
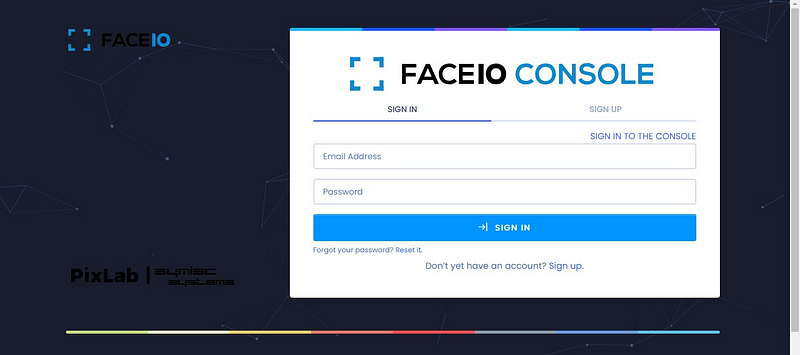
Go to the FACEIO console and sign up for an account.
Retrieve Your Application Public ID and Security Token:
- Log in to your FACEIO account.
- Navigate to the Application Manager in the FACEIO Console.
- Locate your desired application and note down your Application Public ID and security token.
With these prerequisites in place, you’re now ready to integrate FACEIO into your AngularJS application.
Step 3: Import fio.js to Your Angular JS Project
To get started, include the FACEIO JavaScript SDK in your AngularJS project. Add this SDK to your project’s HTML file:
<script src="https://cdn.face.io/js/fio.js"></script>
Step 4: Initialize FACEIO
Create a JavaScript file (e.g., face-auth.js) and initialize FACEIO using your Application Public ID:
const faceio = new Fio({
applicationId: 'YOUR_APPLICATION_PUBLIC_ID'
});
This sets up the FACEIO SDK with your application.
Step 5: Create the Enrollment Process
Next, let’s implement the enrollment process. In your AngularJS application, create a function for enrolling users:
$scope.enrollUser = function () {
faceio.enroll({
payload: {
// Add any additional user data (e.g., Email Address, Name, ID)
},
permissionTimeout: 27, // Wait for user to grant camera access (in seconds)
termsTimeout: 600, // Wait for user to accept Terms of Service (in seconds)
})
.then(userInfo => {
// User successfully enrolled
// You can handle the enrollment success here
})
.catch(errCode => {
// Handle the enrollment error
});
};
In this code:
$scope.enrollUser is an AngularJS function that initiates the enrollment process.
permissionTimeout specifies the time (in seconds) to wait for the user to grant camera access.
termsTimeout specifies the time (in seconds) to wait for the user to accept the Terms of Service.
You can handle enrollment success and error within the then and catch blocks, respectively.
Step 6: Create the Authentication Process
Now, let’s implement the authentication process. In your AngularJS application, create a function for authenticating users:
$scope.authenticateUser = function () {
faceio.authenticate({
permissionTimeout: 27, // Wait for user to grant camera access (in seconds)
termsTimeout: 600, // Wait for user to accept Terms of Service (in seconds)
})
.then(userData => {
// User successfully authenticated
// Handle the authentication success here
})
.catch(errCode => {
// Handle the authentication error
});
};
In this code:
$scope.authenticateUser is an AngularJS function that initiates the authentication process.
permissionTimeout specifies the time (in seconds) to wait for the user to grant camera access.
termsTimeout specifies the time (in seconds) to wait for the user to accept the Terms of Service.
You can handle the authentication success and error within the then and catch blocks.
Step 7: Handle Session Restart
If needed, you can implement the session restart functionality. This allows for another round of calls to enroll() or authenticate() for the same user without reloading the entire HTML page:
$scope.restartSession = function () {
const sessionRestarted = faceio.restartSession();
if (sessionRestarted) {
// Session restarted successfully
// You can perform additional actions if needed
} else {
// Session restart failed
// Handle the failure accordingly
}
};
In this code:
$scope.restartSession is an AngularJS function that calls faceio.restartSession() to request a new user session.
- If the session restart is successful, you can handle it in the first block.
- If the session restart fails, you can handle it in the second block.
Step 8: Define an AngularJS Module and Controller
Finally, define an AngularJS module and controller in your AngularJS application:
var app = angular.module('myApp', []);
app.controller('myCtrl', function ($scope) {
// Define your functions (enrollUser, authenticateUser, restartSession) here
});
Remember to replace ‘YOUR_APPLICATION_PUBLIC_ID’ with your actual Application Public ID.
Privacy by Design
Ensure that your application follows privacy by design principles. Communicate with your users about your privacy practices and give them control over their data. You can incorporate FACEIO’s privacy recommendations into your application’s interface.
Security by Design
Consider the security aspects of your application. You can configure various security settings for your FACEIO implementation through the Application Manager. Review these settings and enable them based on your application’s requirements.
Implementing Webhooks
For real-time updates and notifications about authentication events, set up webhooks in your AngularJS application. These webhooks allow your backend to stay synchronized with FACEIO events. To configure webhooks:
- Log in to your FACEIO account.
- Access the Application Manager.
- Choose your application.
- Navigate to the WEBHOOKS tab.
- Enter your Webhook Endpoint URL to receive events and save your changes.
Handling Webhook Events
Create an HTTP endpoint in your AngularJS application to handle incoming webhook events. The events sent by FACEIO include information like event names, Facial IDs, application IDs, and more. Your application should be capable of processing these events in less than six seconds.
Conclusion
You’ve successfully integrated FACEIO face authentication into your AngularJS application. With FACEIO, you can enhance security, protect user privacy, and provide a frictionless authentication experience. Regularly review and update your security settings to adapt to evolving threats and regulations, ensuring a secure user experience.
By following this step-by-step guide, you’ve taken the first steps toward incorporating facial recognition into your AngularJS application for a more secure and user-friendly authentication process.
Opinions expressed by DZone contributors are their own.
Comments