How to Use AWS IAM Role on AWS EKS PODs
A native-AWS way to attach an IAM role into the Kubernetes POD, without third-party software, reducing latency and improving your EKS security.
Join the DZone community and get the full member experience.
Join For FreeHow It Works
It’s possible to attach an IAM role in a Kubernetes POD without using third-party software, such as kube2iam and kiam. This is thanks to the integration between AWS IAM and Kubernetes ServiceAccount, following the approach of IAM Roles for Service Accounts (IRSA).
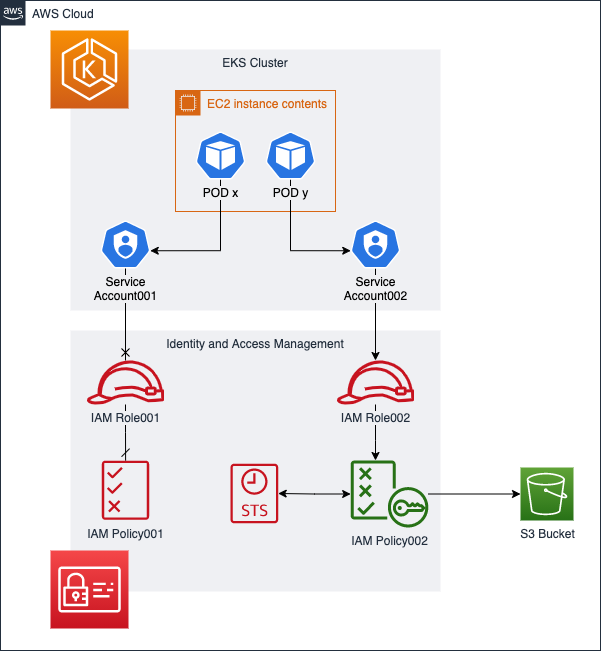
Benefits
There are quite a few benefits of using IRSA with Kubernetes PODs.
- Granular restriction (per cluster, per namespace, etc.).
It’s also possible to not use it. - More flexible than the other tools.
- One less point of failure (maybe a few less).
- Lesser resource consumption.
- More pods per node.
- Latency may reduce by ~50ms.
Especially for the first request. - Prevent issues with caching the credentials.
This software takes a few minutes to update its cache. - Better auditing.
Instead of checking the logs of kube2iam/kiam pods, you can check AWS CloudTrails. - Easier to set up.
- AWS provides full support.
Pre-requirements
There are a few pre-requirements that you’ll need to attempt in order to use the IAM role in a POD.
- An IAM OpenID Connect provider pointing to the AWS EKS OpenID Connect provider URL.
- AWS EKS cluster 1.13 or above.
- A trust relationship between your IAM Role and the OpenID Provider.
Costs
There is no extra cost.
How to Setup
There a few ways to set up, I’ll share how to do it via eksctl and terraform.
I didn’t add eksctl
and terraform
as pre-requirements, since you can do it via AWS Console too.
Both tools eksctl
or terraform
, will set up the exact same thing (except for the IAM Policy that isn't created via eksctl
). These tools will do:
- Create an AWS OpenID Connect provider.
- Link the OIDC provider to the EKS OIDC URL.
- Create an IAM Role.
- Create an IAM Policy (only via terraform).
- Attach the IAM Policy to the IAM Role.
- Set up the Trust Relationship between the IAM Role and the OpenID Connect provider.
- Create a Kubernetes ServiceAccount.
Setting up With eksctl
Using eksctl may be easy for the first time, but it can be trick/hard to automate.
You can follow these scripts/steps:
01-verify_oidc.sh - here we'll just get the EKS OIDC endpoint and check if there is an OpenID Providers created. There is no change in this step.
xxxxxxxxxx
## First of all, check if you already have an OpenID Connect
### List your EKS cluster OIDC URL
aws eks describe-cluster --name <CLUSTER_NAME> --query "cluster.identity.oidc.issuer"
#### Output
https://oidc.eks.<REGION>.amazonaws.com/id/<OIDC_ID>
### List your
aws iam list-open-id-connect-providers
#### The output shouldn't contain any provider with the same <OIDC_ID> listed in the previous command
{
"OpenIDConnectProviderList": []
}
#### OR
{
"OpenIDConnectProviderList": [
{
"Arn": "arn:aws:iam::<ACCOUNT_ID>:oidc-provider/oidc.eks.<REGION>.amazonaws.com/id/<ANOTHER_OIDC_ID>"
}
]
}
02-setting_up.sh - On this step, we'll create an OpenID provider (in case you need it) and then create a Kubernetes Service Account in your EKS Cluster.
xxxxxxxxxx
## Create this only if you don't have an OpenID Connect provider for the cluster
eksctl utils associate-iam-oidc-provider --cluster <CLUSTER_NAME> --approve
## Now it's time to create the AWS IAM Role (<ROLE_NAME>), a Kubernetes ServiceAccount
## and attach an pre-existing IAM Policy (<POLICY_NAME>) into the new role (<ROLE_NAME>)
## The IAM role will be created by the eksctl and attach the pre-existing policy (<POLICY_NAME>) into it.
eksctl create iamserviceaccount \
--cluster=<CLUSTER_NAME> \
--role-name=<ROLE_NAME> \
--namespace=<NAMESPACE> \
--name=<SERVICE_ACCOUNT_NAME> \
--attach-policy-arn=<POLICY_NAME> \
--approve
03-deploy_pod.sh - Last but not least, here is an example of using the IAM Role in a POD by attaching a Service Account.
xxxxxxxxxx
## In the spec, we'll provide the <SERVICE_ACCOUNT_NAME> created previously.
kubectl apply -f- <<EOF
apiVersion: v1
kind: Pod
metadata:
name: amazonlinux
namespace: <NAMESPACE>
spec:
serviceAccountName: <SERVICE_ACCOUNT_NAME>
containers:
- name: amazonlinux
image: amazonlinux
command: [ "sh", "-c", "sleep 8h" ]
EOF
You can check the 04-cleaning.sh in case you want to clean up; just be careful with it.
Setting With Terraform
I created a GitHub repository: LozanoMatheus/eks-oidc. It’s straightforward to adapt to the real scenario.
The main.tf: Here we'll define the TF code to create an OpenID Connect, an IAM Policy, an IAM Role, and a Kubernetes Service Account (plus attach the IAM role to the K8s Service Account):
xxxxxxxxxx
####################
## OpenID Connect ##
####################
data "tls_certificate" "main" {
url = data.terraform_remote_state.eks_cluster.outputs.eks_oidc
}
resource "aws_iam_openid_connect_provider" "main" {
url = data.terraform_remote_state.eks_cluster.outputs.eks_oidc
client_id_list = [
"sts.amazonaws.com",
]
thumbprint_list = [
data.tls_certificate.main.certificates.0.sha1_fingerprint
]
}
################
## IAM Policy ##
################
resource "aws_iam_policy" "main" {
name = var.policy_name
path = "/"
description = "Allow AWS EKS PODs to get a specify S3 Object"
policy = file("${path.module}/policies/s3_policy.json")
}
resource "aws_iam_role" "main" {
name = var.role_name
path = "/"
assume_role_policy = data.template_file.role_trust_relationship.rendered
force_detach_policies = false
}
resource "aws_iam_role_policy_attachment" "main" {
role = aws_iam_role.main.name
policy_arn = aws_iam_policy.main.arn
}
############
## K8s SA ##
############
resource "kubernetes_service_account" "main" {
metadata {
name = var.role_name
namespace = "matheus"
annotations = {
"eks.amazonaws.com/role-arn" = aws_iam_role.main.arn
}
}
automount_service_account_token = true
}
The providers.tf: This code is to configure the TF providers:
xxxxxxxxxx
provider "aws" {
region = var.aws_region
}
terraform {
required_version = "~> 0.12.4"
required_providers {
aws = "~> 2"
tls = "~> 3"
}
backend "s3" {
}
}
provider "kubernetes" {
host = data.terraform_remote_state.eks_cluster.outputs.endpoint ## aws_eks_cluster.main.endpoint
cluster_ca_certificate = base64decode(data.terraform_remote_state.eks_cluster.outputs.ca_cert_base64) ## aws_eks_cluster.main.certificate_authority[0].data
token = data.aws_eks_cluster_auth.cluster.token
load_config_file = false
version = "~> 1.9"
}
The data.tf: This will get the state of your EKS cluster (in case you create it in another TF), get the EKS Cluster ID, and configure the IAM Policy (to allow you to use this policy inside of the EKS Cluster):
xxxxxxxxxx
#################
## EKS Cluster ##
#################
data "terraform_remote_state" "eks_cluster" {
# ...
}
data "aws_eks_cluster_auth" "cluster" {
name = element(concat(data.terraform_remote_state.eks_cluster.outputs.*.id, list("")), 0) ## aws_eks_cluster.main.id
}
##################
## IAM Policies ##
##################
data "template_file" "role_trust_relationship" {
template = file("${path.module}/policies/role_trust_relationship.json.tmpl")
vars = {
oidc_arn = aws_iam_openid_connect_provider.main.arn
oidc_url = aws_iam_openid_connect_provider.main.url
k8s_namespace = var.k8s_namespace
role_name = var.role_name
}
}
The vars.tf: This is the TF file that contains the variables used by the TF. It will define the AWS Region (eu-west-1
), set the Kubernetes namespace that the IAM Policy can be used, the IAM Role name, and the IAM Policy name.
x
variable "aws_region" {
type = string
default = "eu-west-1"
}
variable "k8s_namespace" {
default = "tips-and-tricks"
}
variable "role_name" {
default = "s3-get-object-iam-role001"
}
variable "policy_name" {
default = "s3-get-object-iam-policy001"
}
The policies/s3_policy.json: This is the policy that will allow the interaction between the K8s POD and AWS APIs. In this case, I'm allowing the POD to get objects from a specific S3 bucket in a specific path:
xxxxxxxxxx
{
"Version": "2012-10-17",
"Statement": [{
"Effect": "Allow",
"Action": [
"s3:GetObject"
],
"Resource": [
"arn:aws:s3:::aws-s3-example/file001"
]
}]
}
The policies/role_trust_relationship.json.tmpl: This is a template file and it will allow the POD to use the IAM role:
xxxxxxxxxx
{
"Version": "2012-10-17",
"Statement": [{
"Effect": "Allow",
"Principal": {
"Federated": "${oidc_arn}"
},
"Action": "sts:AssumeRoleWithWebIdentity",
"Condition": {
"StringEquals": {
"${oidc_url}:sub": "system:serviceaccount:${k8s_namespace}:${role_name}",
"${oidc_url}:aud": "sts.amazonaws.com"
}
}
}]
}
Now you can create all the resources:
x
## Planning
terraform plan
## Deploying
terraform apply
Published at DZone with permission of Matheus Lozano. See the original article here.
Opinions expressed by DZone contributors are their own.
Comments