How to Read JSON Files in Java Using the Google Gson Library
This tutorial blog focuses on teaching how to read the JSON files using the Google Gson library with different sample JSON files.
Join the DZone community and get the full member experience.
Join For FreeJSON files are commonly used these days for sending data to applications. Be it a web application, an API, or a mobile application, JSON is used by almost every team as it is lightweight and self-describing.
Due to its high popularity and wide usage, it is important to understand and know what JSON is, its features, its different data types, file formats, etc. In this blog, we will be learning about JSON, its features, data types, and file formats. We will then continue to learn to read JSON files in Java using the Google Gson library.
Table of Contents
- What is JSON?
- Features of JSON
- JSON Data Types
- JSON Syntax Rules
- Understanding the JSON file content
- How to read JSON files in Java using the Google Gson library
What Is JSON?
JSON stands for JavaScript Object Notation and is an open-standard data interchange format known for being self-describing and lightweight. It was derived from JavaScript and was originally specified by Douglas Crockford.
JSON is both easy to read and write and is also language-independent. It supports data structures such as arrays and objects, allowing for flexible data organization. It is widely used by software teams for storing and transporting data. It is often used when data is sent from a server to web pages; the RESTful APIs use JSON files for sending and receiving data over HTTP.
Features of JSON
The following are some of the key features of JSON.
- Simple and easy to understand: JSON is self-describing which makes it simple and easy to read and write. It helps in efficiently transferring data over the internet.
- Language independent: JSON uses a text-based format that can be parsed and easily used with multiple programming languages. This comparatively makes it much faster than other text-based structured data.
- Support for complex data types: JSON supports multiple data types including
Strings
,numbers
,boolean
,objects
,arrays
, andnull
. - Interoperability: Since JSON originated from JavaScript, it integrates seamlessly with JavaScript-based applications, making it highly suitable for web development.
JSON Data Types
As just listed, the following data types are supported by JSON:
String
Number
Boolean
Objects
Arrays
Null
JSON Syntax Rules
The following are important syntax rules for JSON
- Data is in name/value pairs.
- Data is separated by commas.
- Curly braces hold objects.
- Square brackets hold arrays.
- JSON does not support comments.
- The JSON file should be saved with the extension .json.
Understanding the JSON File Content
There are multiple ways in which the JSON file content can be designed as follows:
- It can be an object that has an Array holding multiple objects.
- It can be an Array with multiple Objects.
- It can only be an Object.
- It can be an array with an Object holding another array and so on.
JSON Objects
Here is an example of a JSON file that has only a JSON object as its content.
{
"carname": "Ferrari",
"makeyear": 2024,
"engine": "F1V12",
"estimatedprice": "450K USD"
}
The data is stored in a key/value pair within the curly brackets “{ }
” in the JSON object.
Named JSON Objects
JSON allows naming the JSON objects. This can be done by naming the JSON object as shown in the example below:
{
"employees": [
{
"id": "1",
"activeEmployee": true,
"designation": "Sr. Manager",
"bankdetails": {
"bankname": "Axis Bank Ltd",
"branch": "Mumbai",
"ifsc": "UTIV9089",
"accountno": 2646768
}
},
{
"id": "1",
"activeEmployee": true,
"designation": "Sr. Manager",
"bankdetails": {
"bankname": "Axis Bank Ltd",
"branch": "Mumbai",
"ifsc": "UTIV9089",
"accountno": 2646768
}
}
]
}
The above JSON file holds the employee details. The employee bank details are stored in a JSON object that is named “bankdetails”
. This can also be referred to as the “bankdetails”
key holding the value for the details of the bank.
"bankdetails": {
"bankname": "Axis Bank Ltd",
"branch": "Mumbai",
"ifsc": "UTIV9089",
"accountno": 2646768
}
JSON Arrays
A JSON array stores data within a JSON file as a block enclosed in square brackets, [ ]
.
Here is an example of a JSON file that has JSON Array as its content.
[
{
"numberrange": 5,
"name": "Dylan Hood",
"phone": "1-216-578-5381",
"email": "sollicitudin@aol.com",
"country": "Belgium",
"alphanumeric": "QKU41NZC5PB"
},
{
"numberrange": 7,
"name": "Jeremy Joyce",
"phone": "1-751-217-3163",
"email": "in.faucibus@aol.com",
"country": "Indonesia",
"alphanumeric": "YBO34KQQ8HO"
}
]
In the above example, there is a JSON array holding two objects within it. The data within square brackets [ ]
is an Array and the data within curly brackets { }
are objects.
Named JSON Array
JSON allows naming the arrays. So, we can provide names to the JSON array for easy recognition and readability. The following is an example of a JSON file showing the employee details in an array named “employees”
.
{
"employees": [
{
"name": "John",
"email": "john@gmail.com",
"age": 26,
"designation": "QA"
},
{
"name": "Dennis",
"email": "dennis.c@gmail.com",
"age": 30,
"designation": "Developer"
},
{
"name": "Elizabeth",
"email": "elizb@gmail.com",
"age": 24,
"designation": "Secretary"
},
{
"name": "Steve",
"email": "s.deff@gmail.com",
"age": 29,
"designation": "Test Architect"
}
]
}
How to Read Json Files in Java Using the Google Gson Library
JSON files are simple, easy, and lightweight, as we read in the earlier section of the blog about the different file content types a JSON file holds. Let’s now focus on how to read the JSON file using the Google Gson library.
What Is Google Gson?
Gson, developed by Google, is a Java library that enables the conversion of Java objects to JSON format and can also parse JSON strings to create equivalent Java objects. It is an open-source library available on GitHub that is hugely popular with 23.4k stars and 4.3k forks.
Getting Started
We will be creating a Maven project and adding the following dependency in the pom.xml file.
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.11.0</version>
</dependency>
Additionally, we will be adding the Lombok dependency that will allow us to create POJOs on runtime and avoid writing boilerplate codes. We will also add the Hamcrest dependency that will help us in assertions.
<dependency>
<groupId>org.hamcrest</groupId>
<artifactId>hamcrest-all</artifactId>
<version>1.3</version>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.34</version>
<scope>provided</scope>
</dependency>
This completes the project setup, let’s now delve into the code and learn to read the JSON files using the Google Gson library.
How to Read a JSON File That Has Only a JSON Object
Let’s begin with a simple JSON file that has a JSON object. The following file will be used in the demo and we will be printing the values in the console after reading the file.
- Filename: cardetails.json:
{
"carname": "Ferrari",
"makeyear": 2024,
"engine": "F1V12",
"estimatedprice": "450K USD"
}
Let’s create a new Java class ReadJsonObject
and add the following code to the main
method.
public class ReadCarDetails {
public static void main (String[] args) {
Gson gson = new Gson ();
try (Reader reader = new FileReader (System.getProperty ("user.dir") + "/src/test/resources/cardetails.json")) {
CarDetails carDetails = gson.fromJson (reader, CarDetails.class);
System.out.println (carDetails);
} catch (IOException e) {
throw new Error("Error reading JSON file");
}
}
}
Code Walkthrough
We will be using the Reader
class of Java to read the file cardetails.json that is stored in the “src/test/resources” folder. The System.getProperty(“user.dir”)
will navigate to the working directory (i.e., the root folder of the project) and then using the path “src/test/resources/” we will be navigating to the actual file cardetails.json.
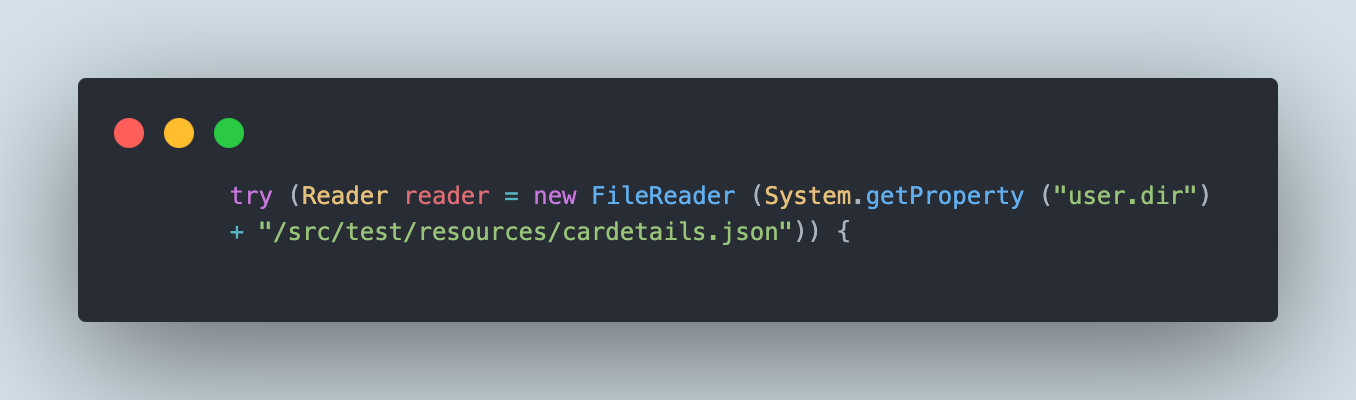
We will be using the Try with resources
statement as it ensures that each resource is closed at the end of the statement. It auto closes the resource so we don’t have to handle it specifically.
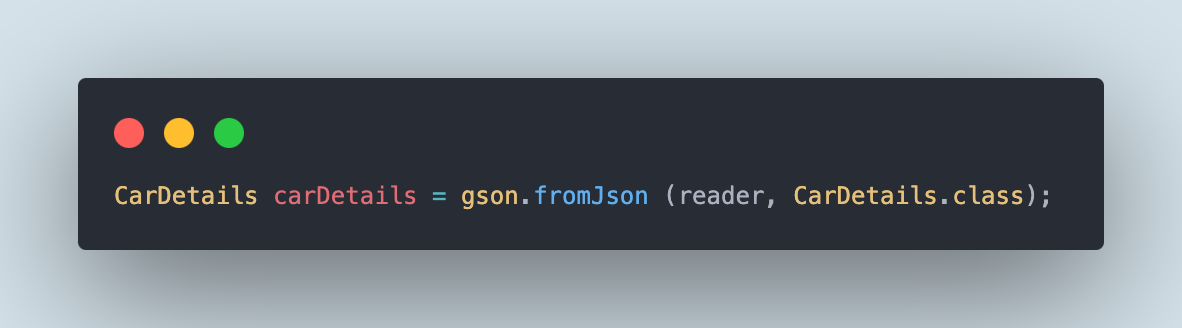
The fromJson()
method from the Gson class will be used to read the JSON file. It accepts two parameters: the first one is the JSON reader and the second one is the POJO class.
The POJO class contains all the corresponding key names of the file. A new class CarDetails.java
has been created to handle the POJO.
@ToString
@Getter
public class CarDetails {
private String carname;
private int makeyear;
private String engine;
private String estimatedprice;
}
Notice that there are no Getters and Setters in this POJO class. It is because we are using Lombok and have placed the annotation @Getter
over the class declaration. This @Getter
annotation ensures that the Getter methods will be created on runtime for further use. Similarly, the @ToString
annotation will convert the object to the string so we can view the actual string values in the output.
Finally, we will be printing all the values in the console after reading the JSON file.
Output
The following output of the program is printed in the console.
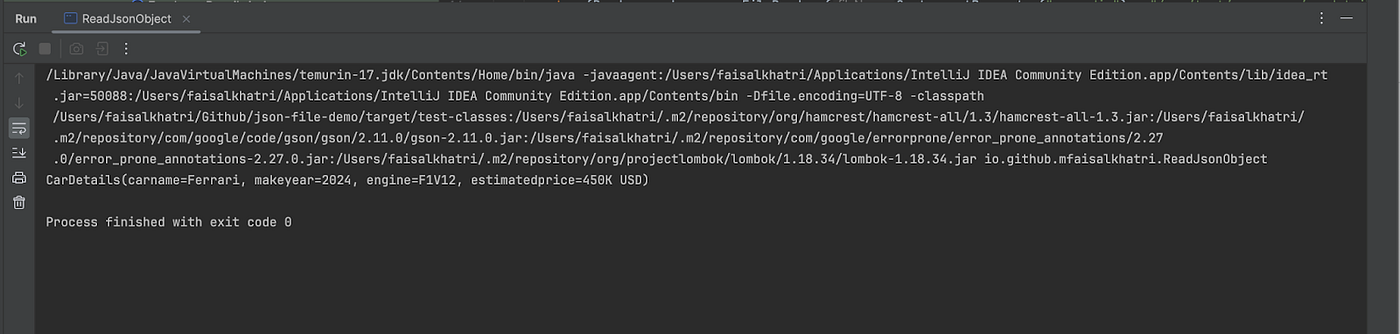
The file details with the key and its respective values are printed in the console correctly as expected.
How to Read a JSON File That Has JSON Arrays
A JSON file can hold data in JSON Arrays that can have multiple JSON Objects. There are multiple approaches using which the JSON file with JSON Arrays can be read.
The following three approaches will be demonstrated for reading the JSON file with JSON Array:
- Reading the JSON Arrays using the
TypeToken
class of Gson library - Reading the JSON Arrays using Java
List
- Reading the JSON Arrays using Java
Arrays
Reading the JSON Arrays Using the TypeToken Class of Gson Library
Let’s take an example JSON file holding the customer details inside a JSON Array with multiple JSON objects.
- Filename: customerdetails.json:
[
{
"customerid": 5,
"name": "Dylan Hood",
"phone": "1-216-578-5381",
"email": "sollicitudin@aol.com",
"country": "Belgium",
"Coupon": true
},
{
"customerid": 7,
"name": "Jeremy Joyce",
"phone": "1-751-217-3163",
"email": "in.faucibus@aol.com",
"country": "Indonesia",
"Coupon": true
},
{
"customerid": 8,
"name": "Kyle Dominguez",
"phone": "(835) 147-8401",
"email": "pede.blandit@icloud.net",
"country": "Vietnam",
"Coupon": true
},
{
"customerid": 19,
"name": "Libby Nash",
"phone": "1-145-851-9979",
"email": "integer@outlook.com",
"country": "Spain",
"Coupon": false
},
{
"numberrange": 21,
"name": "Willow Graves",
"phone": "1-356-817-7211",
"email": "ipsum.suspendisse@outlook.edu",
"country": "Singapore",
"Coupon": false
}
]
A POJO class needs to be created that will help in deserializing the JSON file and help us in parsing and getting the values from the file.
@Getter
@ToString
public class CustomerDetails {
private int customerid;
private String name;
private String phone;
private String email;
private String country;
private boolean coupon ;
}
The Lombok library will be used here as it removes the need to write the boilerplate code for the Getter methods. With Lombok, we just need to place the @Getter
annotation over the POJO class.
Next, we would write the code to read the file by creating a new class ReadCustomerDetails.java
.
public class ReadCustomerDetails {
public static void main (String[] args) {
Gson gson = new Gson ();
try (
Reader reader = new FileReader (
System.getProperty ("user.dir") + "/src/test/resources/customerdetails.json")) {
Type listCustomerDetailsType = new TypeToken<List<CustomerDetails>> () {
}.getType ();
List<CustomerDetails> customerDetailsList = gson.fromJson (reader, listCustomerDetailsType);
System.out.println ("Printing all the customer details from the file: " + customerDetailsList);
System.out.println (
"Printing the customer details from the third Json Object from file: " + customerDetailsList.get (2));
System.out.println (
"Printing the name of the customer from second Json Object: " + customerDetailsList.get (1)
.getName ());
System.out.println (
"Printing the email of the customer from third Json Object: " + customerDetailsList.get (2)
.getEmail ());
System.out.println (
"Printing the Coupon details of customer from the third Json object: " + customerDetailsList.get (2)
.isCoupon ());
MatcherAssert.assertThat (customerDetailsList.get (3)
.getCountry (), equalTo ("Spain"));
} catch (IOException e) {
throw new Error ("Error reading JSON file");
}
}
}
Code Walkthrough
The Reader
class of Java is used to read the JSON file, “customerdetails.json,” which is stored in the “src/test/resources/” folder.
The System.getProperty(“user.dir”)
is used to retrieve the current working directory of the Java process and then the relative path “src/test/resources/customerdetails.json” is supplied.
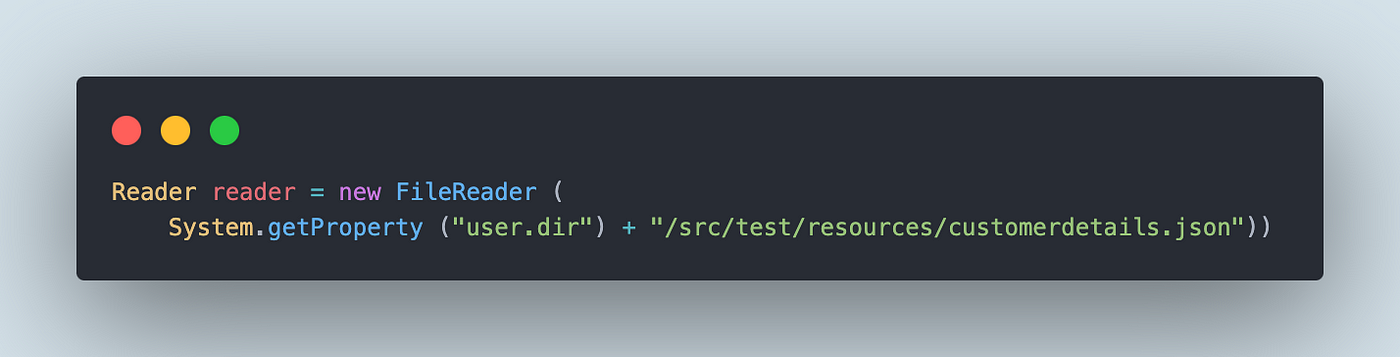
The reader
object created in the first line is used further to read the JSON file’s content.
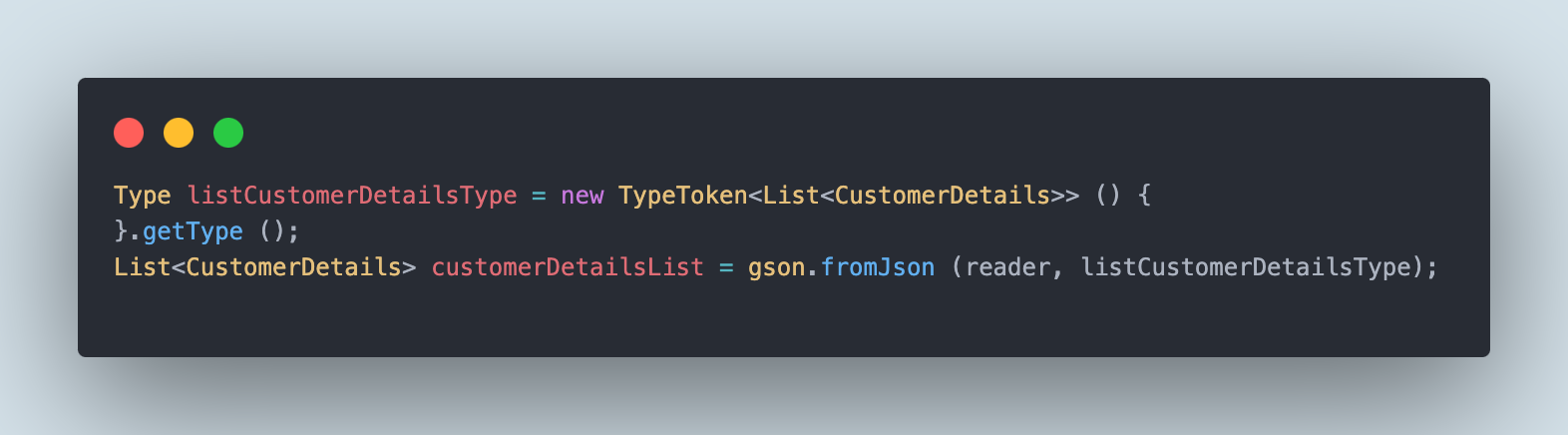
The Type
is a generic type descriptor in Java that is used for storing type information at runtime. It is specifically more useful while dealing with complex generic types. The TypeToken
class from the Gson library is used for getting the generic type information for List<CustomerDetails>
.
The TypeToken
is a helper class from Gson that is used for converting JSON objects to Java and vice versa. The .getType()
method fetches the Type
object and provides information to Gson to correctly deserialize JSON into List<CustomerDetails>
.
Next, the fromJson()
method of the Gson library is used to parse the JSON data into the Java object. It accepts two parameters: the first one is the reader
object that points to the JSON data in the file and the second one is the listCustomerDetailsType
that provides the Type
information to Gson so it can deserialize the JSON into List<CustomerDetails>
.
The customerDetailsList
will hold all of the CustomerDetails
objects. Each of the objects will be populated with data from the JSON file. The customerDetailsList
can then be further used for printing the JSON file values in the console.
The following code will print all the customer details in the console from all the JSON objects in the file.
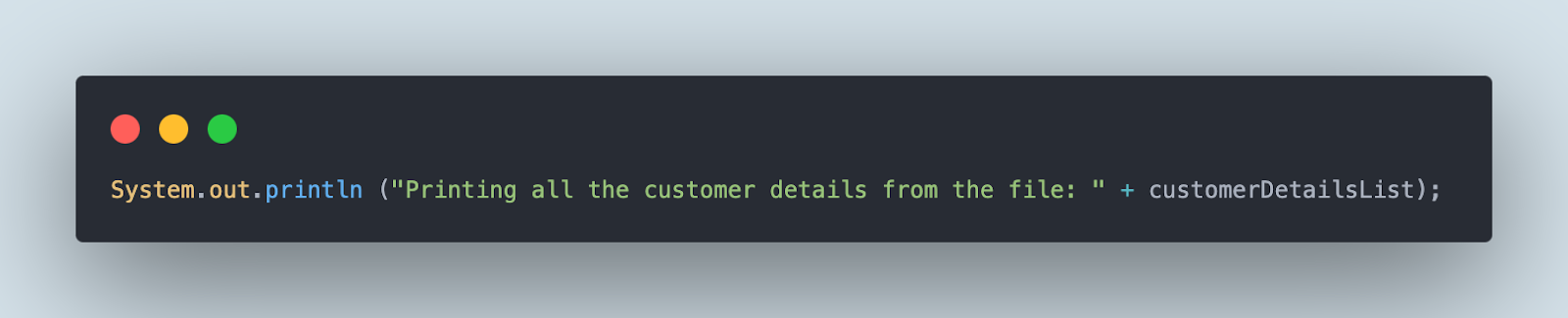
The following line of code will print all the customer details in the console from the third JSON object in the file. This is done by calling the get()
method with index “2
”(since arrays start with 0, hence index 2 is used to get the third object).
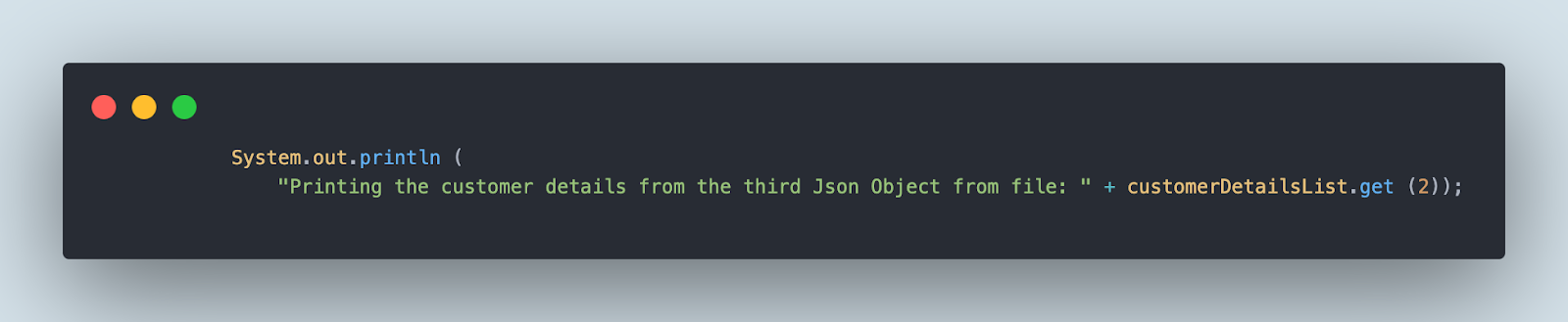
All the getter methods are generated on runtime using Lombok, hence we just need to call the object index using the get()
method and then simply call the Getter
method to get the values from the file.
The following line of code will print the customer name
from the second JSON object in the file.
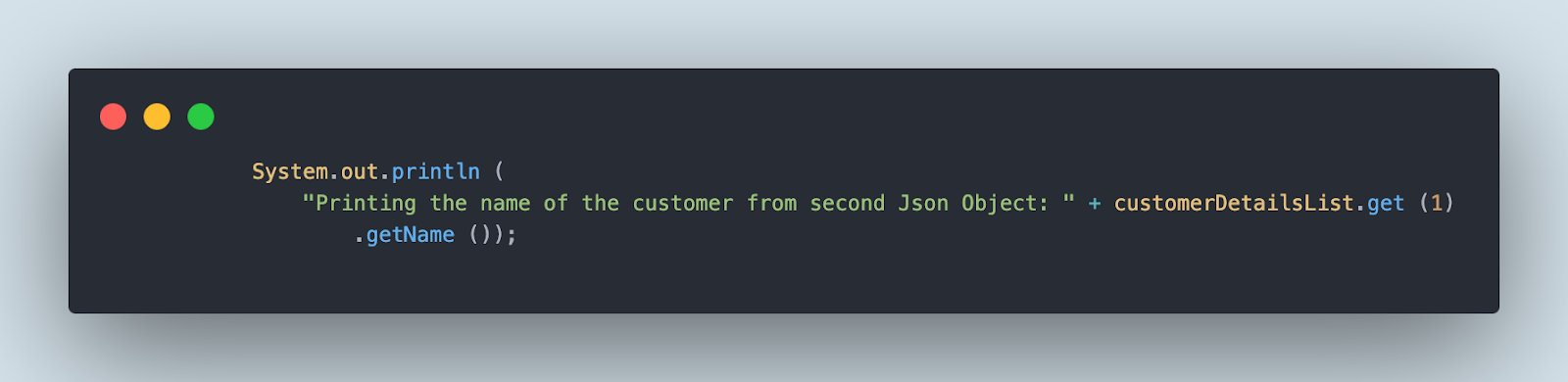
The following line of code will print the email
and coupon
details from the third JSON object in the file.
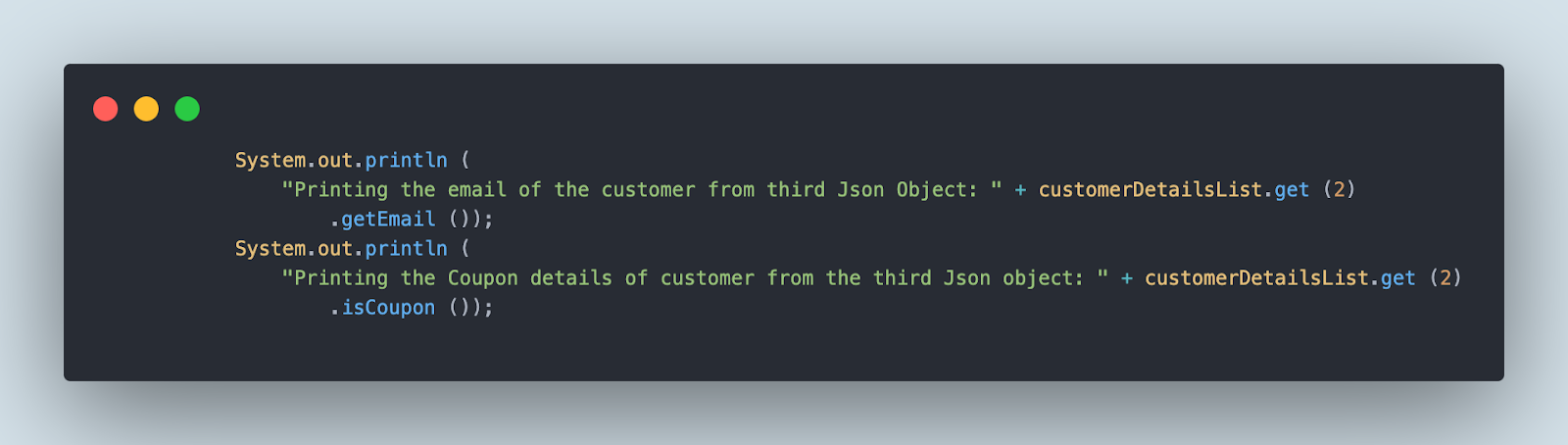
We would be performing an assertion to check that the correct country name
is fetched from the file for the customer from the fourth JSON object.
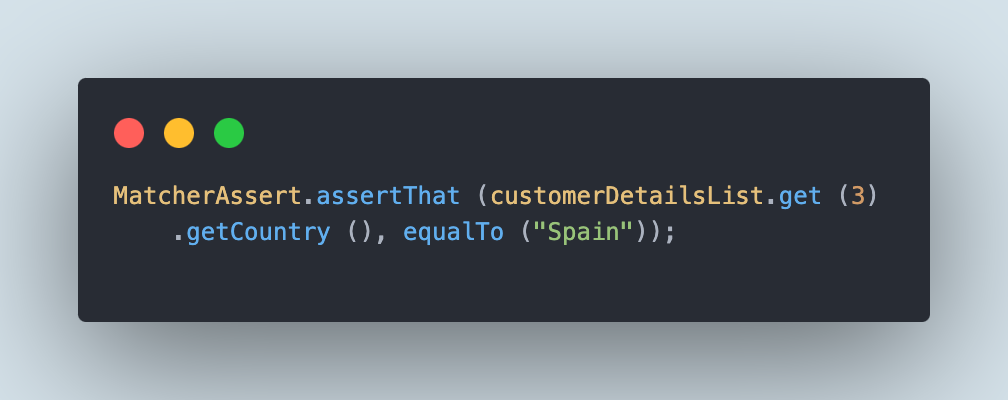
This assertion is written using the assertThat()
method from the Hamcrest library.
Output
The following output of the program is printed in the console:
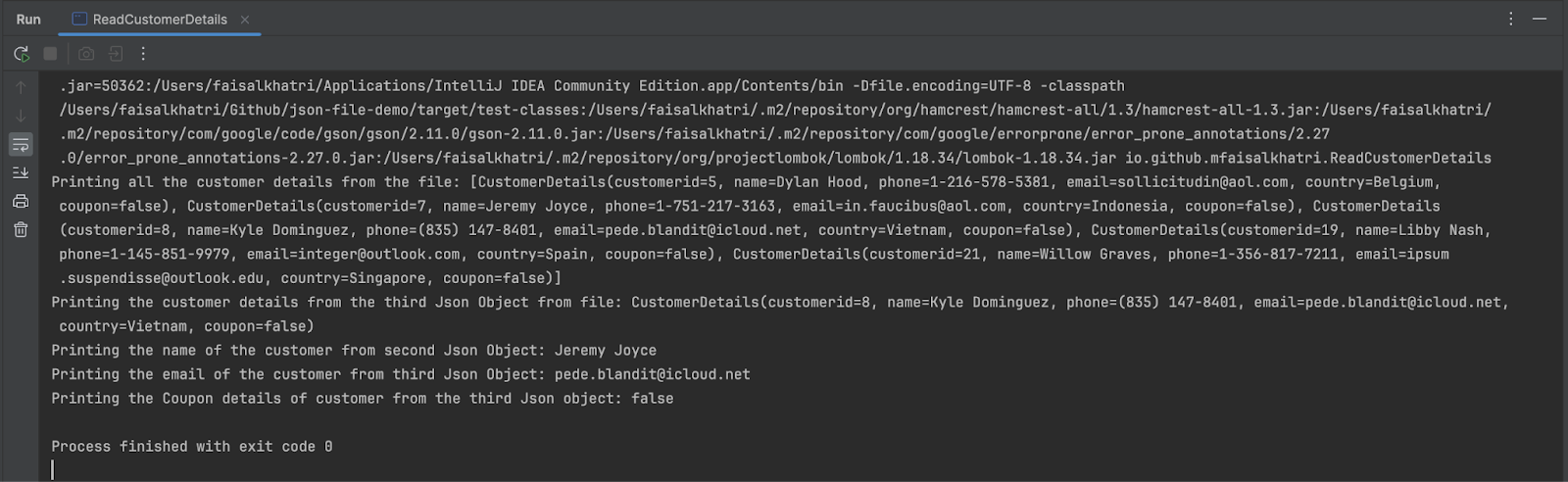
In the first line, all the customer details are printed. Next, customer details from the third JSON object are printed following which the name of the customer from the second JSON object is printed.
Finally, the email and the coupon details from the third JSON object are printed.
Reading the JSON Arrays Using Java List
In the next example, we will be reading a JSON file holding the employee details in an array of multiple JSON objects. This is a JSON Array with the name “employees”
.
- Filename: employeedetails.json
{
"employees": [
{
"name": "John",
"email": "john@gmail.com",
"age": 26,
"designation": "QA"
},
{
"name": "Dennis",
"email": "dennis.c@gmail.com",
"age": 30,
"designation": "Developer"
},
{
"name": "Elizabeth",
"email": "elizb@gmail.com",
"age": 24,
"designation": "Secretary"
},
{
"name": "Steve",
"email": "s.deff@gmail.com",
"age": 29,
"designation": "Test Architect"
}
]
}
The POJO class , EmployeeDetails.java
, will help us in deserializing and parsing this JSON file.
@Getter
@ToString
public class EmployeeDetails {
private List<Employees> employees;
}
The POJO is pretty simple: it holds the list of all the employees that will be handled using another POJO class , Employees.java
.
@Getter
@ToString
public class Employees {
private String name;
private String email;
private int age;
private String designation;
}
The Employees
class has all the fields available in the JSON object. The next step is to create a new class ReadEmployeeDetailsAsList.java
that will read and parse the JSON file and print the output of the JSON file in the console.
public class ReadEmployeeDetailsAsList {
public static void main (String[] args) {
Gson gson = new Gson ();
try (
Reader reader = new FileReader (
System.getProperty ("user.dir") + "/src/test/resources/employeedetails.json")) {
EmployeeDetails employeeDetails = gson.fromJson (reader, EmployeeDetails.class);
System.out.println ("Printing all the Employee Details: " + employeeDetails);
System.out.println (
"Printing the Employee Name from the first Json object: " + employeeDetails.getEmployees ()
.get (0)
.getName ());
System.out.println (
"Printing the Employee Designation from the second Json Object: " + employeeDetails.getEmployees ()
.get (1)
.getDesignation ());
MatcherAssert.assertThat (employeeDetails.getEmployees ()
.get (2)
.getEmail (), equalTo ("elizb@gmail.com"));
} catch (IOException e) {
throw new Error ("Error reading JSON file");
}
}
}
Code Walkthrough
An instance of the Reader
class is created implementing the FileReader
class from Java that will read the contents of the JSON file.
Next, the fromJson()
method of the Gson library is used for parsing the JSON data into the Java object. There are two parameters supplied to this method: the first one is the reader object that points to the JSON data in the file and the second one is the specific target type for deserialization.
Gson will use the EmployeeDetails
class to create an instance of the said class and populate it with the “employees” data from the JSON file.
The following line of code will print all the employee JSON objects from the file:
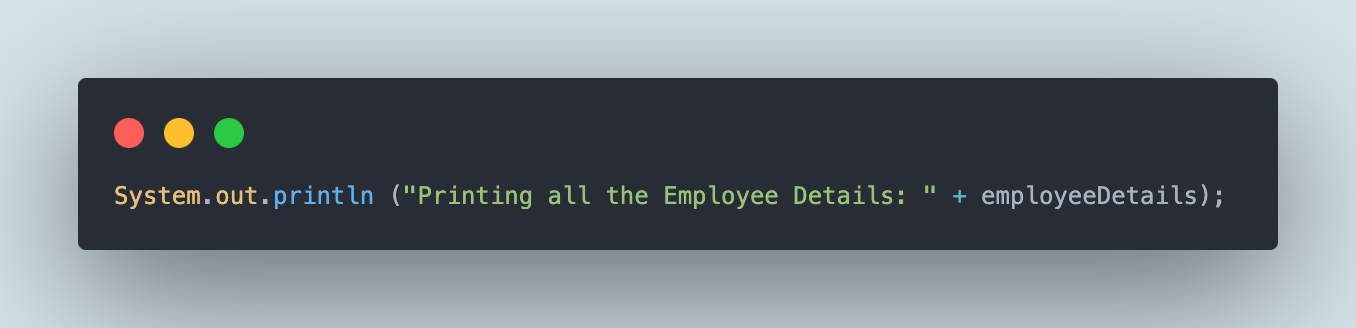
The following first print statement will print the employee name from the first JSON object whereas the second print statement will print the employee designation from the second JSON object respectively.
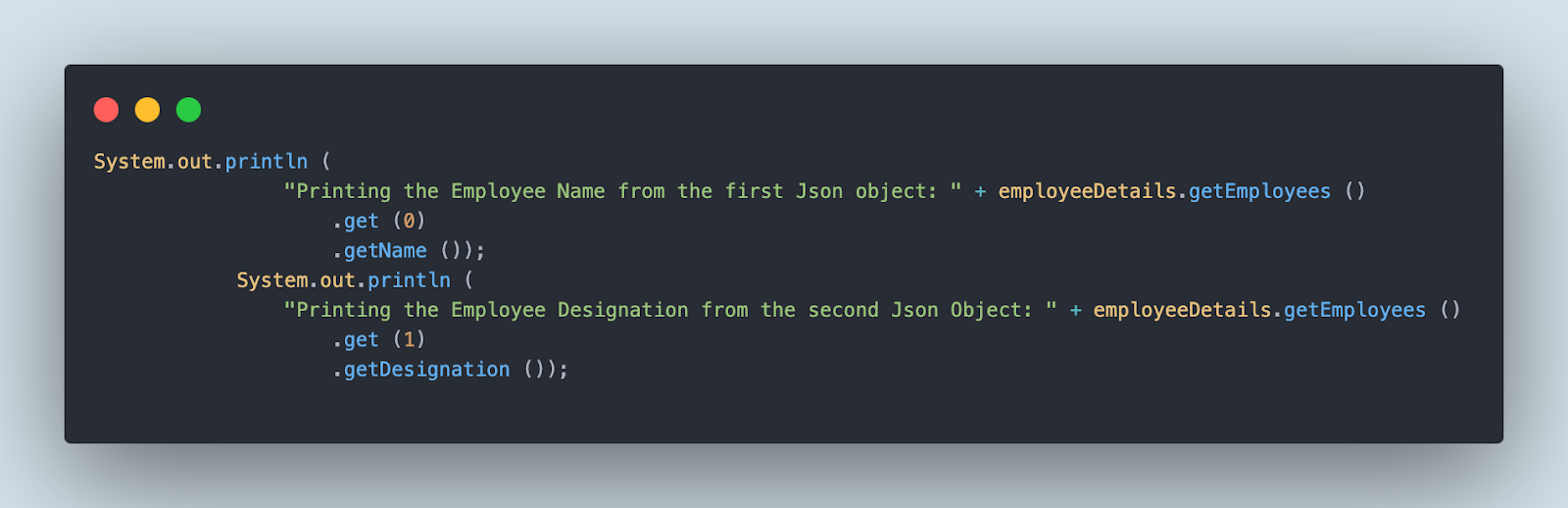
We can also perform assertion of the values using the Hamcrest library by fetching the value from the JSON file and matching it with the expected value as shown in the below screenshot.
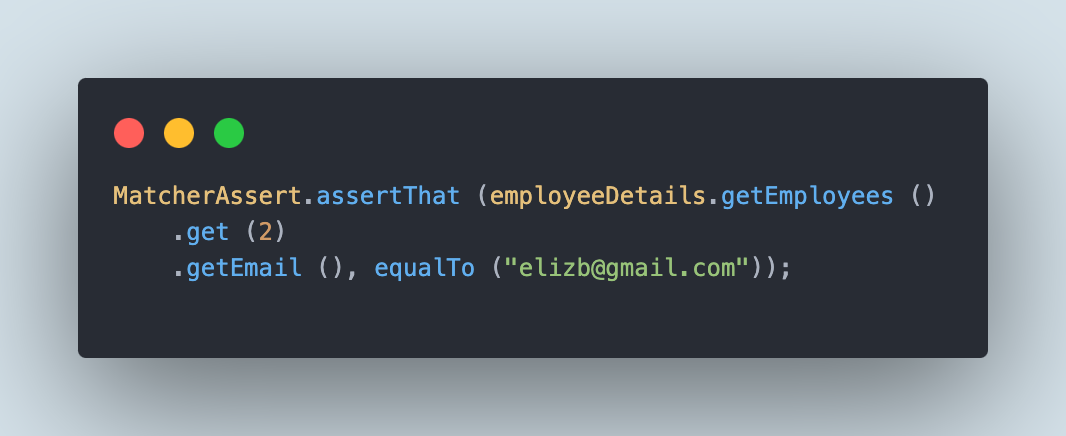
The above assert statement will get the employee email from the third JSON object and check that it equals to "elizb@gmail.com"
.
Output
The following is the output of the code printed in the console after the code is run.
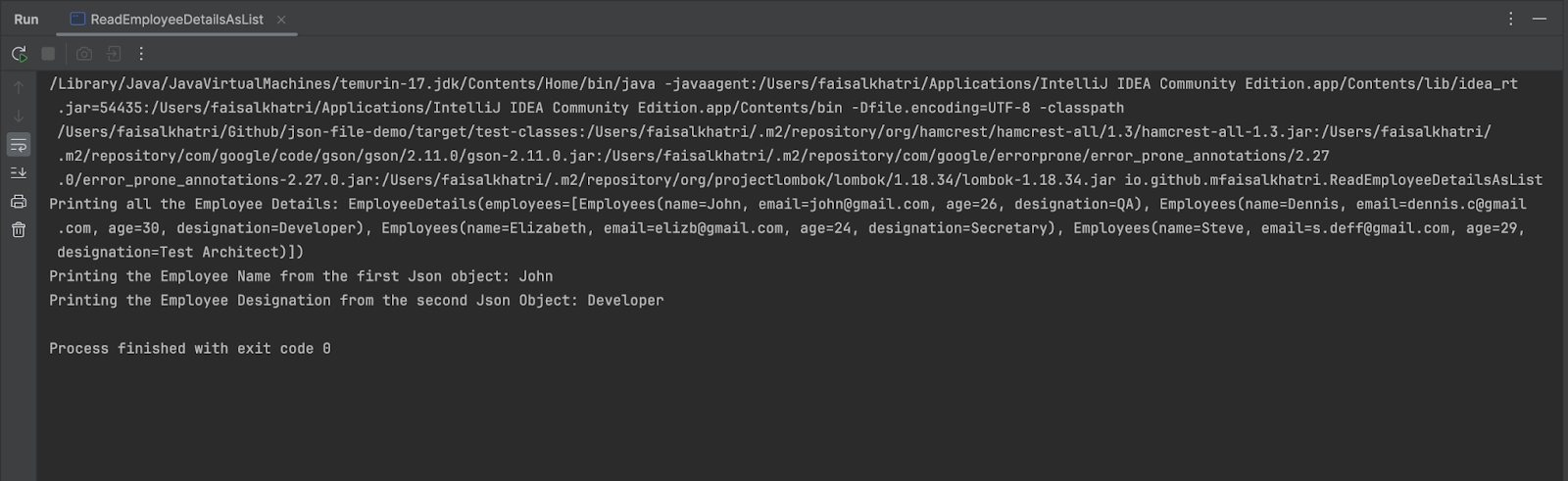
It can be seen in the console that all the details of the employees are printed first. Next, the Employee Name — “John”
from the first JSON object is printed successfully. On the last line of the console, the Employee Designation = “Developer”
, from the second JSON object is printed successfully.
Reading the JSON Arrays Using Java Arrays
Another way to read the JSON file with Arrays is using the Java Arrays. In this section, we will be using the same EmployeeDetails.json file and reading it using Java Arrays.
- Filename: employeedetails.json
{
"employees": [
{
"name": "John",
"email": "john@gmail.com",
"age": 26,
"designation": "QA"
},
{
"name": "Dennis",
"email": "dennis.c@gmail.com",
"age": 30,
"designation": "Developer"
},
{
"name": "Elizabeth",
"email": "elizb@gmail.com",
"age": 24,
"designation": "Secretary"
},
{
"name": "Steve",
"email": "s.deff@gmail.com",
"age": 29,
"designation": "Test Architect"
}
]
}
Let’s create a POJO class , EmployeeDetails.java
, with a Java Array to parse the JSON file.
@Getter
@ToString
public class EmployeeDetailsAsArray {
private Employees[] employees;
}
We would be using the existing POJO class Employees.java
for mapping the JSON fields.
@Getter
@ToString
public class Employees {
private String name;
private String email;
private int age;
private String designation;
}
The next step is to create a new class ReadEmployeeDetailsAsList.java
that will read and parse the JSON file and print the output of the file in the console.
public class ReadEmployeeDetailsAsArray {
public static void main (String[] args) {
Gson gson = new Gson ();
try (
Reader reader = new FileReader (
System.getProperty ("user.dir") + "/src/test/resources/employeedetails.json")) {
EmployeeDetailsAsArray employeeDetails = gson.fromJson (reader, EmployeeDetailsAsArray.class);
System.out.println ("Printing all the Employee Details: " + employeeDetails);
System.out.println (
"Printing the Employee Name from the first Json object: " + employeeDetails.getEmployees ().length);
System.out.println (employeeDetails.getEmployees ()[1].getName ());
} catch (IOException e) {
throw new Error ("Error reading JSON file");
}
}
}
Code Walkthrough
After reading the file using the Reader
class of Java, we will be using the reader object and passing it as a parameter to the fromJson()
method of Gson
class.
The second parameter of the fromJson()
method is the EmployeeDetailsAsArray.class
that will create an instance of the said class and populate it with the “employees” data from the JSON file as an array.
The following line of code will print all the JSON file values in the console.
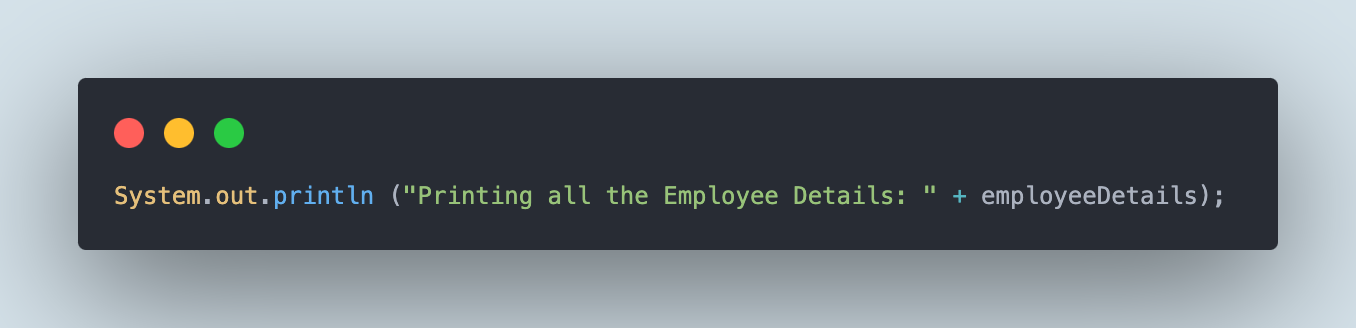
Next, the following line of code will print the total number of JSON objects in the file.
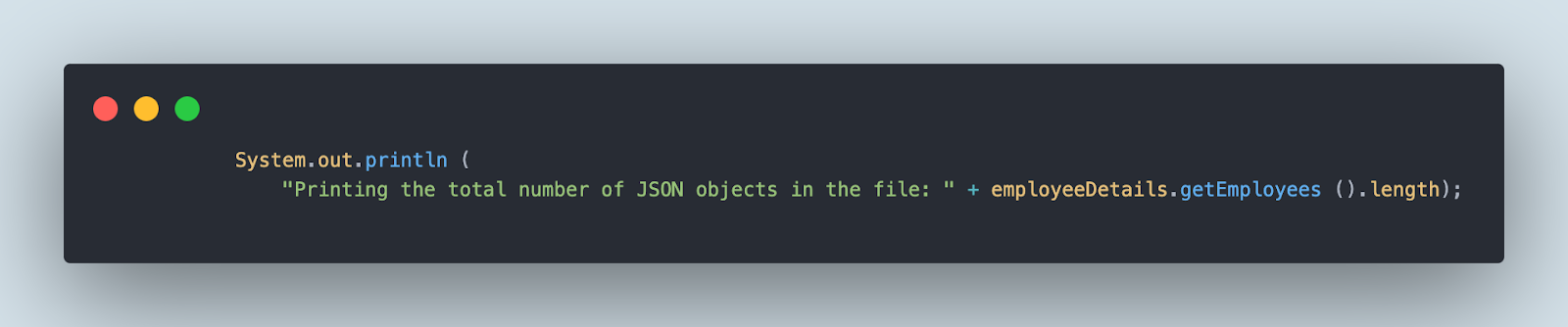
Now, to print the employee name
from the second JSON object, we need to write the following line of code. It uses “[]” to get the required array object value.
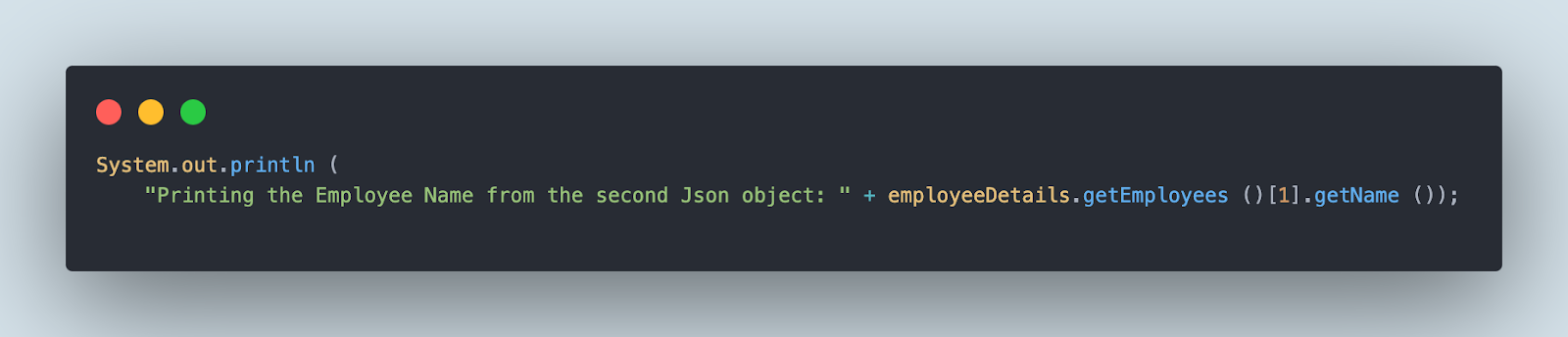
If we talk about readability, using Java List
or using the TypeToken
class to read the file is much simpler as it allows us to easily navigate to the JSON file objects. However, using Arrays, we will have to check for the corresponding index to get the details from the file which becomes a bit complex.
Output
The following output is printed in the console after running the code.
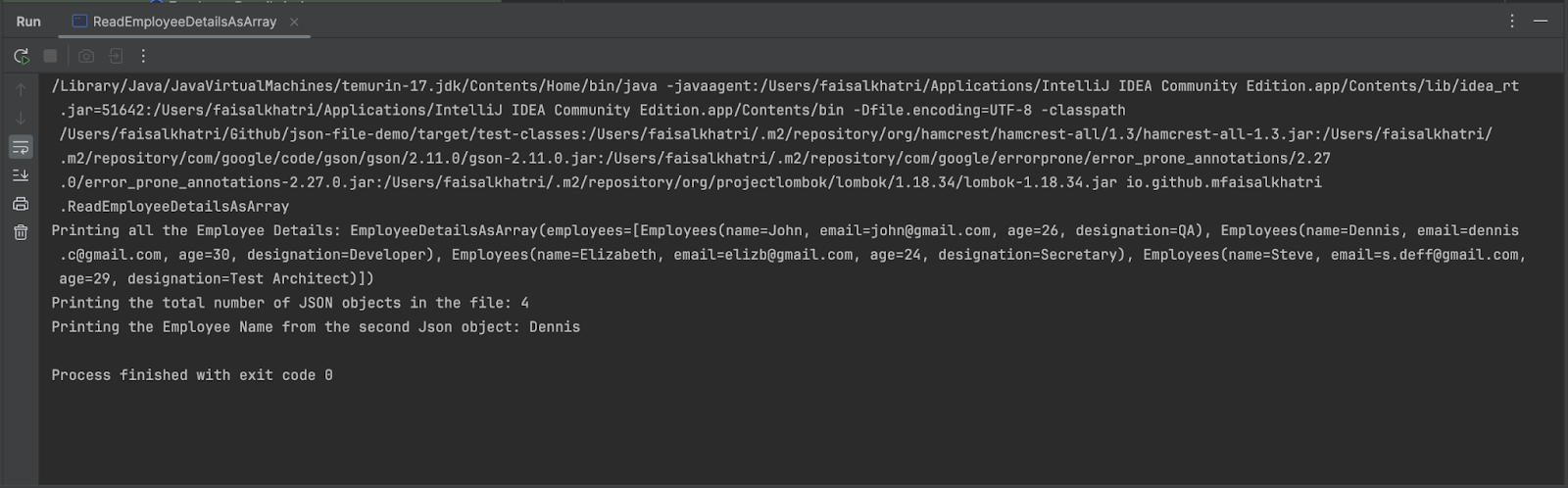
All the employee details are printed first, following the total number of JSON objects in the file, and finally the employee name “Dennis
” from the second JSON object.
How to Read a JSON File That Has a JSON Object Within a JSON Object
A JSON file can also have JSON objects that have another JSON object within it. Let’s consider one such example of a file, passengerdata.json below:
- Filename : passengerdata.json
{
"passengerdetails": [
{
"id": 1,
"name": "John Doe",
"activeTraveller": true,
"origin": "Paris",
"destination": "Frankfurt",
"ticketdetails": {
"airlines": "Saudi Airlines",
"pnr": "PFJF45G",
"date": "29/10/2024",
"ticketamt": 45000
}
},
{
"id": 2,
"name": "Richard Dave",
"activeTraveller": true,
"origin": "Mumbai",
"destination": "Dubai",
"ticketdetails": {
"airlines": "Emirates",
"pnr": "PCG78Y",
"date": "31/10/2024",
"ticketamt": 26000
}
}
]
}
The passengerdata.json file has multiple objects of passenger details
. Each Passenger detail has an object of “ticket details
” that has multiple fields in it.
In order to read this file, we will need to create three POJO classes. The first POJO class will be for parsing the main JSON Array that holds the “passengerdata
”.
We will be using Java List to parse the JSON arrays.
@Getter
@ToString
public class PassengerData {
private List<PassengerDetails> passengerdetails;
}
The second POJO will be for parsing the “passengerdetails
” and reading all its fields.
@Getter
@ToString
public class PassengerDetails {
private int id;
private String name;
private boolean activeTraveller;
private String origin;
private String destination;
private TicketDetails ticketdetails;
}
Note: The name of the class and the name of the JSON Array should be the same. In our case, the name of the JSON array is “passengerdetails
” hence the POJO class is named as “PassengerDetails
”.
An important point to note in this class is that, since the “ticketdetails
” object is inside every object of the “passengerdetails
”, hence we will also place the TicketDetails
POJO class inside the PassengerDetails
class.
The third POJO will be used for parsing the “ticketdetails
” object and reading its fields.
@Getter
@ToString
public class TicketDetails {
private String airlines;
private String pnr;
private String date;
private int ticketamt;
}
After creating the POJO classes, we can move straight to writing the actual code for parsing and reading the contents of the “passengerdata.json” file.
Let’s create a new Java class, ReadPassengerData.json
.
public class ReadPassengerData {
public static void main (String[] args) {
Gson gson = new Gson ();
try (
Reader reader = new FileReader (
System.getProperty ("user.dir") + "/src/test/resources/passengerdata.json")) {
PassengerData passengerData = gson.fromJson (reader, PassengerData.class);
System.out.println ("Printing all the Passenger Data: " + passengerData);
System.out.println (
"Printing the Passenger Name from the first Json object: " + passengerData.getPassengerdetails ()
.get (0)
.getName ());
System.out.println (
"Printing the Ticket Details of the first Json object: " + passengerData.getPassengerdetails ()
.get (0)
.getTicketdetails ());
MatcherAssert.assertThat (passengerData.getPassengerdetails ()
.get (1)
.getDestination (), equalTo ("Dubai"));
} catch (IOException e) {
throw new Error ("Error reading JSON file");
}
}
}
Code Walkthrough
The approach to reading the file remains the same as we learned through this blog in the earlier sections. We would be calling the fromJson()
method of the Gson library where the reader object, an object of the Reader
class of Java is supplied after reading the JSON file.
In the second parameter, the PassengerData.class
object, is supplied that will create an instance of the said class and populate it with the “passengerdetails
” data from the JSON file.
We would be printing the details in the console by writing the code using Java print statements. The following print statement will print all the JSON file data in the console.
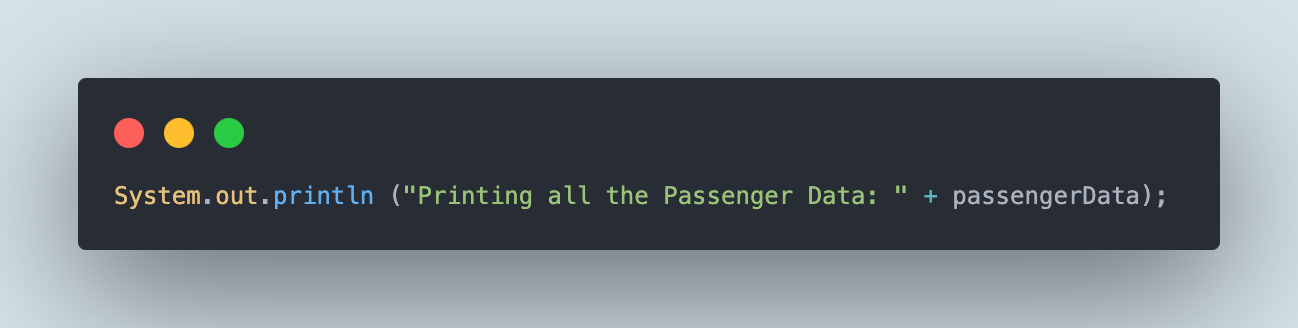
The following print statement will print the passenger name from the first JSON object.
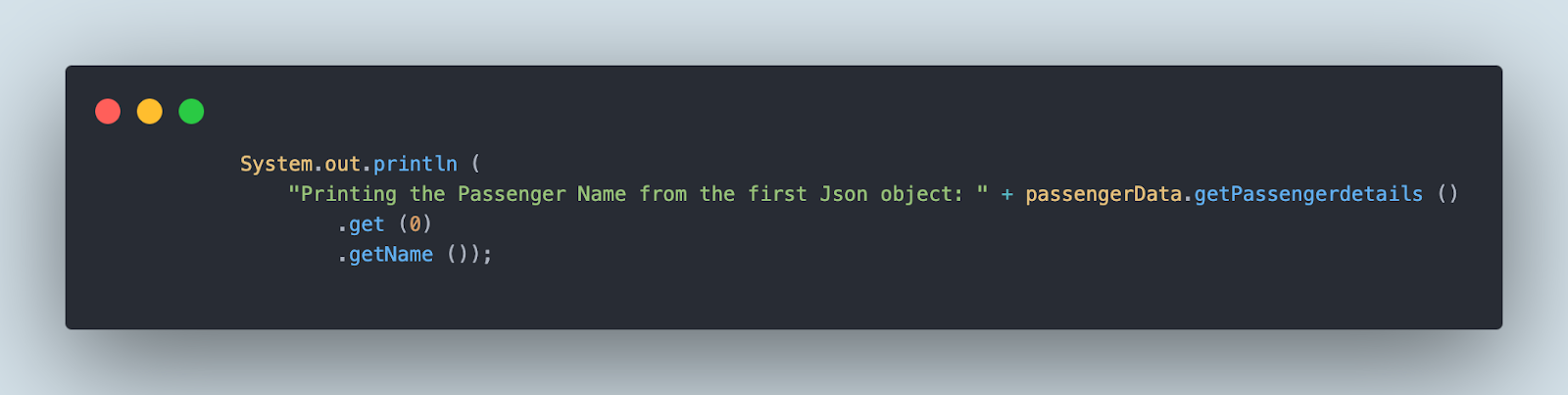
The following print statement will check that the destination of the second passenger in the ticket details is “Dubai”
.
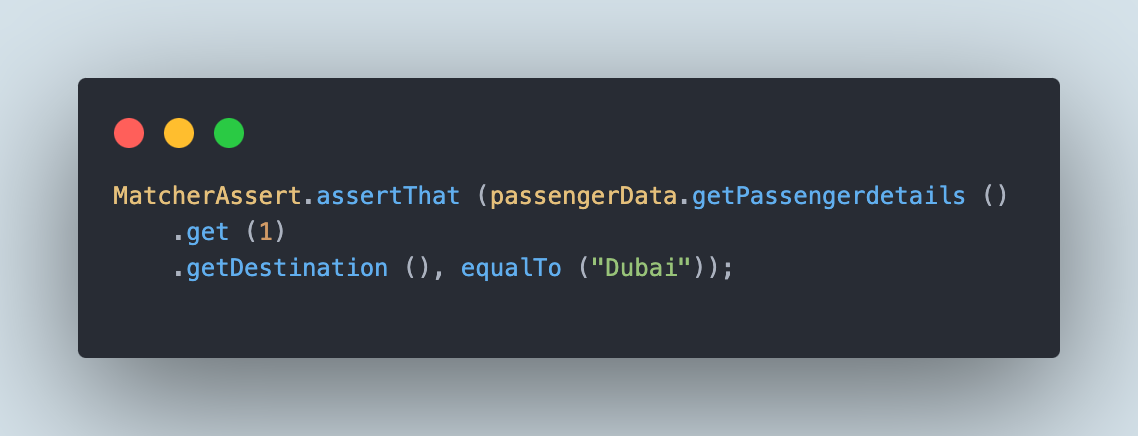
Likewise, we can use the instance of the PassengerData
class and read the other details in the JSON file.
Output
Let’s execute the code and check for the details printed in the console.
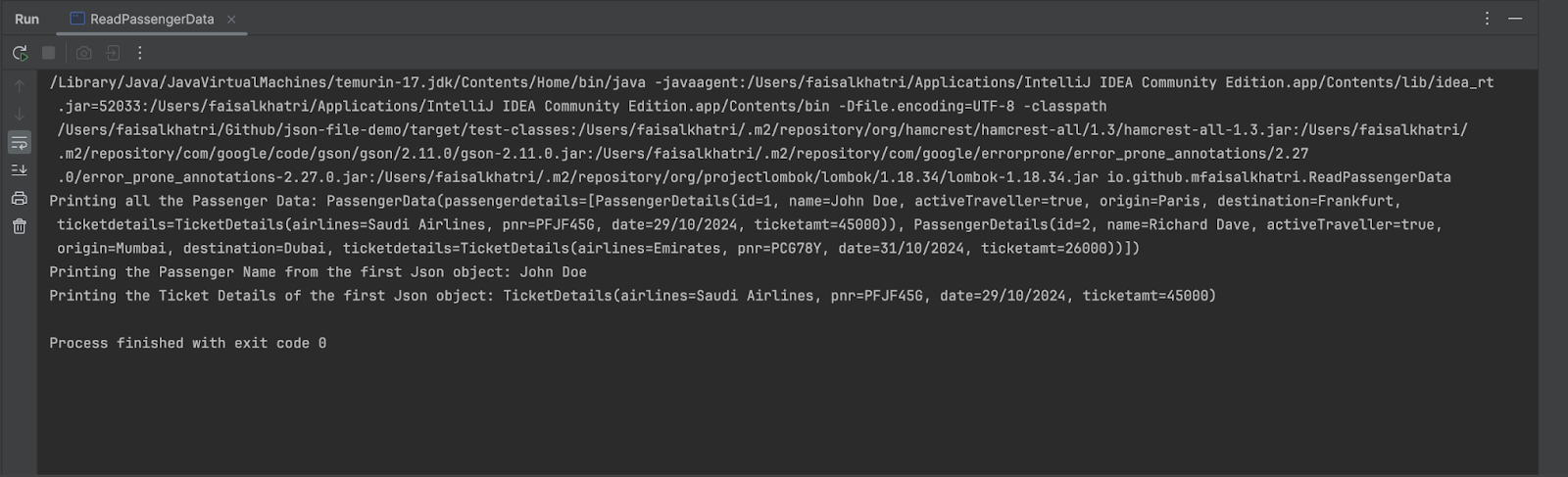
In the first line, all the passenger details from the JSON file are printed including the ticket details. In the second line, the passenger name, i.e., “John Doe”, from the first JSON object is printed.
In the last line, all the details of the ticket are printed for the first passenger. Lastly, as the assertion passed for checking the destination of the second passenger, the execution did not throw any exception.
Summary
The Google Gson library allows us to parse and read the JSON file easily. This library comes in handy in case we want to set up configurations in the test automation/development projects using the JSON file. Gson is a very lightweight and useful library that allows us to easily read the file with minimum code.
I have used this library in my test automation projects for reading the test framework configurations for Web, Mobile, and API testing. I hope this tutorial blog gave a fair idea about using Gson to parse and read the different JSON file contents with different approaches.
Happy testing!
Published at DZone with permission of Faisal Khatri. See the original article here.
Opinions expressed by DZone contributors are their own.
Comments