Useful Decorators and Functions in Python's Functools
The most important functions from the Functools module of Python are total ordering, reduce, and partial. Here's how they work and why they're so important.
Join the DZone community and get the full member experience.
Join For FreeThe Functools module of Python is a collection of Higher-Order Functions. A Higher-Order Function is one that:
- Takes function as a parameter.
- Return a function as its return value from another function.
Here are the three most important functions or decorators from the Functools module of Python and why each is so important.
Total Ordering
#total_ordering is a decorator that is used mostly used for Object Comparison. To create an Orderable class, we can define methods like __gt__()
, __ge__()
,__lt__()
, __le__()
,__eq__()
which corresponds to ( >, >=, <, <=, ==).
Python is smart enough to understand that it’s a tedious task to define all these methods in a class, to overcome that apart from __eq__
only one method needs to be defined manually, the rest will be provided by Python automatically, so, all in all, we need to define two methods one must be __eq__
and the other can be anyone out of the collection of magic methods.
By defining magic methods in a class and its implementation, this implementation will work by default in the collection of objects as well, whenever functions like sorted, sort, max, min, reduce, etc. is being used on Collection. Let’s create a list of employees:
The current implementation of magic methods is on employee salary; the sorting will take place based on employee salary in Ascending Order.
Reverse=True
Max / Min
Upon removal of magic methods from the class, in this case, __gt__
method and trying to use sorted/max/min any function without a key will result in a runtime error, which clearly indicates that functions were trying to mutually compare and sort the objects
Reduce
The reduce() function reduces a sequence to a single value. This function takes two parameters, function and sequence (list), then it applies the function to every element of an iterable until the single value is returned. For example:
The way reduce works is, it takes the first two parameters from the list which are 1 and 2, then (1 + 2) = 3. Then the result of the previous operation is applied to the next parameter, which is 3, it results in (3 + 3) = 6 and so on, the diagram summarizes it:
In the example lambda is used, which is also a function without a name, which means instead of lambda, any function can be passed since reduce is a higher-order function, above example can be re-written as:
The reduce() function also works with Custom Objects, in the Employee example, we declared a list called employees, using reduce function to find the employee with maximum salary.
In the employees list, 200 was the maximum salary, but in this example, the Employee class defines the __gt__
method, so the reduce function is finding the employee with maximum salary by default. Let’s redeclare the list and find the employee with max-age using reduce function. Of course, max, min can be used in the example, but the idea here is to explore the reduce() function.
Partial
The partial() function is an important functional programming concept, it brings code re-usability. The idea is to create new functions from the existing function by fixing few function arguments.
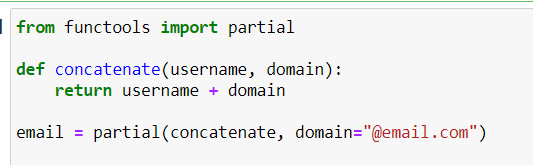
By using the partial function above, we are telling Python to fix the value of the second parameter, but do not fix the value of the first parameter.
If you print ‘email’ it’s a partial function:
This "email" function can be passed to other functions as a parameter. In our employees list example, let’s generate the email-ids of all the employees with their names.
Opinions expressed by DZone contributors are their own.
Comments