Embracing the Zen of Python: A Simple Guide to Mastering Pythonic Programming
Let's explore the Zen of Python with code examples to improve your skills as a Python developer. Join us on this journey!
Join the DZone community and get the full member experience.
Join For FreePython is a versatile and user-friendly programming language that has taken the coding world by storm. Its simplistic syntax and extensive libraries have made it the go-to choice for both beginners and experienced developers. Python’s popularity can be attributed not only to its technical merits but also to its unique guiding principles known as the “Zen of Python.”
The “Zen of Python” is a collection of aphorisms that encapsulate the guiding philosophy for writing code in Python. Authored by Tim Peters, a renowned software developer and one of the earliest contributors to Python, these principles were first introduced in a mysterious way.
In the early days of Python, the Zen of Python was included as an Easter egg in the language. To reveal it, users had to type import this
in the Python interpreter. This enigmatic and playful approach added an element of curiosity to the already thriving Python community, leading to much speculation about its origins.
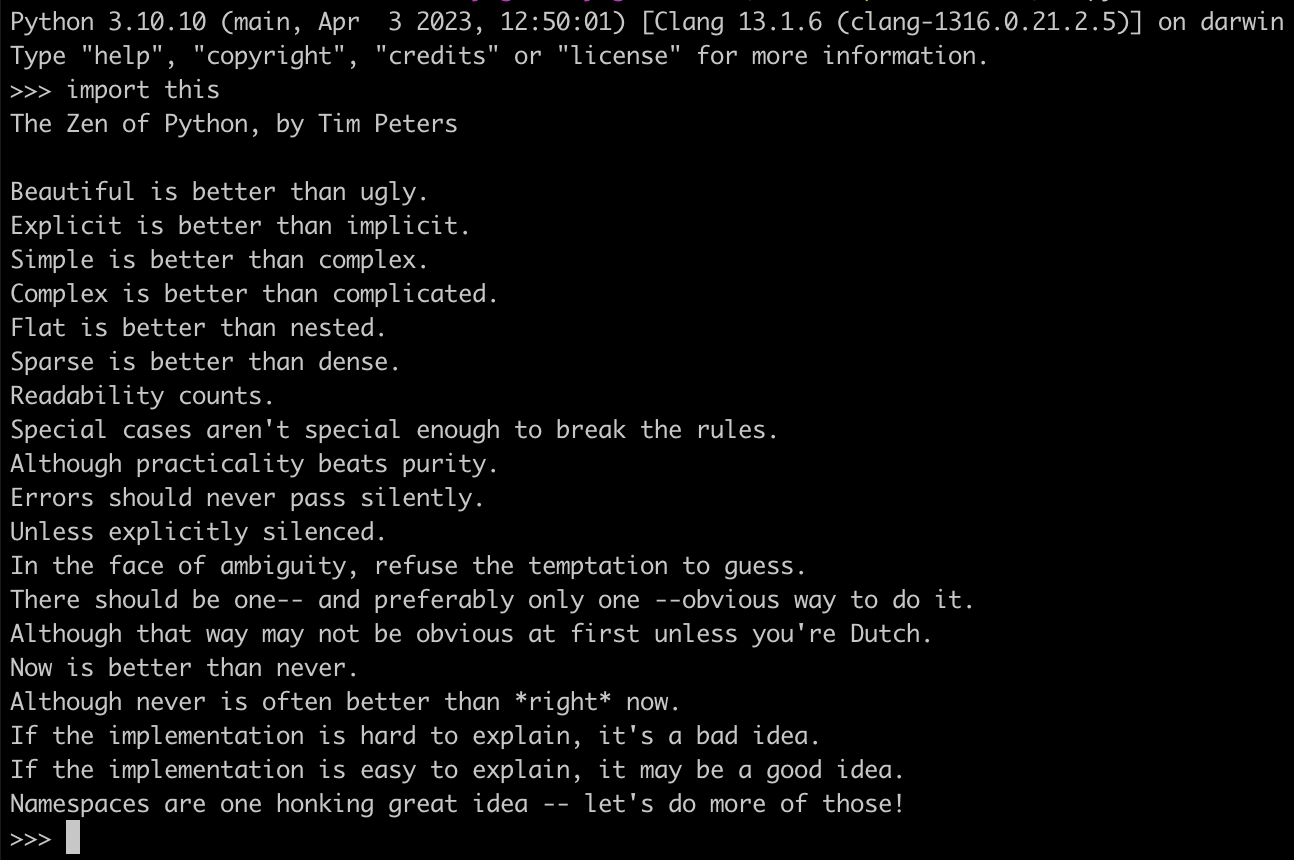
The Zen of Python was finally revealed in its entirety, and it has since become a cherished set of guidelines for Python developers. It captures the essence of Python’s design philosophy, emphasizing simplicity, elegance, and readability as paramount virtues in code.
What Is Zen?
The term “Zen” originates from Zen Buddhism, a school of Mahayana Buddhism that emphasizes meditation, intuition, and a direct understanding of reality. In the context of Python, the Zen of Python embodies a similar philosophy, promoting simplicity, clarity, and harmony in code.
The Zen of Python serves as a guiding compass for Python developers, providing a set of aphorisms that encourage a mindful and thoughtful approach to coding. By adhering to these principles, Python programmers strive to create code that not only accomplishes its intended purpose but also conveys a sense of elegance and beauty.
In the sections that follow, we will explore each of the Zen of Python principles in detail, providing code examples to illustrate their practical application. By the end of this blog post, you will have a deeper understanding of how to harness the power of Zen in your Python code, propelling you towards becoming a more proficient and mindful Python developer. So, let’s embark on this enlightening quest to unravel the Zen of Python!
1. Beautiful Is Better Than Ugly
Python places a strong emphasis on code aesthetics. Writing beautiful code not only enhances its visual appeal but also makes it easier for others to comprehend and collaborate. As a beginner, take pride in crafting clean and well-structured code, and you’ll quickly appreciate the artistry in Python programming.
# Non-Pythonic code
def add_numbers(a, b): return a + b
# Pythonic code
def add_numbers(a, b):
return a + b
2. Explicit Is Better Than Implicit
In Python, as in life, clarity is essential. Be explicit in your code, leaving no room for ambiguity. Clearly state your intentions, define variables explicitly, and avoid magic numbers or hidden behaviors. This approach ensures that your code is easy to understand and less prone to errors.
# Implicit
def calculate_area(radius):
return 3.14 * radius ** 2
# Explicit
from math import pi
def calculate_area(radius):
return pi * radius ** 2
3. Simple Is Better Than Complex
Simplicity is a core principle of Python. Embrace straightforward solutions to solve problems, avoiding unnecessary complexities. As a beginner, you’ll find that Python’s simplicity empowers you to express ideas more effectively and efficiently.
# Complex code
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n - 1)
# Simple code
def factorial(n):
result = 1
for i in range(1, n + 1):
result *= i
return result
4. Complex Is Better Than Complicated
Wait! Didn’t we just say, “Simple is better than complex”!?
While simplicity is favored, complex problems may require complex solutions. However, complexity should not equate to obscurity. Aim to create clear and well-structured code, even when dealing with intricate challenges.
# Complicated
def calculate_fibonacci(n):
if n == 0:
return 0
elif n == 1:
return 1
else:
a, b = 0, 1
for i in range(n - 1):
a, b = b, a + b
return b
# Complex but not complicated
def calculate_fibonacci(n):
if n == 0:
return 0
elif n == 1:
return 1
else:
return calculate_fibonacci(n - 1) + calculate_fibonacci(n - 2)
5. Flat Is Better Than Nested
Code readability and simplicity play pivotal roles in effective programming. Instead of delving into intricate layers of indentation and nested structures, break down complex tasks into manageable components. The result is code that is more comprehensible, maintainable, and easier to collaborate on.
# Nested
def process_data(data):
result = []
for item in data:
if item > 0:
if item % 2 == 0:
result.append(item)
return result
# Flat
def process_data(data):
result = []
for item in data:
if item > 0 and item % 2 == 0:
result.append(item)
return result
6. Sparse Is Better Than Dense
It’s tempting to pack a lot of functionality into a single line, and it might impress your non-coder friends, but it definitely won’t impress your colleagues. Spread out code is easier to understand, and don’t forget beautiful is better than ugly.
# Dense
def process_data(data):result=[];for item in data:if item>0:result.append(item);return result
# Sparse
def process_data(data):
result = []
for item in data:
if item > 0:
result.append(item)
return result
7. Readability Counts
As developers, we read code more often than we write it. Therefore, prioritize code readability by adhering to consistent formatting, using meaningful variable names, and following Python’s style guide (PEP 8). Readable code is easier to maintain, debug, and collaborate on.
# Hard to read
def p(d):
r = []
for i in d:
if i > 0:
r.append(i)
return r
# Easy to read
def process_data(data):
result = []
for item in data:
if item > 0:
result.append(item)
return result
8. Special Cases Aren’t Special Enough to Break the Rules
There are well-defined best practices and rules when it comes to writing good Python code. Stick to them.
9. Although Practicality Beats Purity
This contradicts #8. Well, kind of. If your code is easier to follow but deviates from the earlier principle, go for it! Beginners have a tough time finding the balance between practicality and purity, but don’t worry; it gets better with experience.
10. Errors Should Never Pass Silently
Always opt for comprehensive error handling. By catching and handling errors, you can prevent unexpected behavior and make your code more robust.
# Errors pass silently
def divide(a, b):
try:
return a / b
except:
pass
# Errors are handled explicitly
def divide(a, b):
try:
return a / b
except ZeroDivisionError:
print("Error: Cannot divide by zero.")
11. Unless Explicitly Silenced
In certain scenarios, there might be a deliberate decision to overlook potential errors triggered by your program. In such cases, the recommended approach is to purposefully suppress these errors by addressing them explicitly within your code.
# Explicitly silence exceptions
def divide(a, b):
try:
return a / b
except ZeroDivisionError:
pass
12. In the Face of Ambiguity, Refuse the Temptation To Guess
Don’t attempt random solutions with the singular purpose of making your code “work.” Instead, dig deeper into the problem, debug, and understand what is causing it. Then, and only then, work on fixing it. Don’t guess!
13. There Should Be One — And Preferably Only One — Obvious Way To Do It
Python’s motto encourages consistency and standardization. Whenever possible, use the idiomatic Pythonic way to solve problems. This uniformity simplifies code reviews, enhances collaboration, and ensures a smooth experience for fellow developers.
14. Although That Way May Not Be Obvious at First Unless You’re Dutch
Tim Peters introduces a dose of humor that captures the essence of this principle. In a playful nod to Guido van Rossum, the creator of Python, Peters quips that only the “Zen Master” himself might possess an encyclopedic knowledge of all the seemingly obvious ways to implement solutions in Python.
15. Now Is Better Than Never
Don’t hesitate to start coding! Embrace the “now” mindset and dive into projects. As a beginner, getting hands-on experience is crucial for learning and improving your coding skills.
16. Although Never Is Often Better Than Right Now
While being proactive is important, rushing into coding without a clear plan can lead to suboptimal solutions and increased technical debt. Take the time to understand the problem and plan your approach before diving into writing code
17. If the Implementation Is Hard To Explain, It’s a Bad Idea
Strive for code that is easy to explain and understand. If you find yourself struggling to articulate your code’s logic, it might be a sign that it needs simplification. Aim for code that communicates its purpose effectively.
# Hard to explain implementation (using bitwise operators)
def is_even(n):
return n & 1 == 0
# Easy to explain implementation (using modulo operator)
def is_even(n):
return n % 2 == 0
18. If the Implementation Is Easy To Explain, It May Be a Good Idea
Conversely, if your code’s logic is evident and easy to explain, you’re on the right track. Python’s elegance lies in its ability to make complex tasks appear simple.
19. Namespaces Are One Honking Great Idea — Let’s Do More of Those!
Python’s use of namespaces allows for organizing code logically and reducing naming conflicts. Embrace modular design and create reusable functions and classes, keeping your codebase organized and maintainable. However, also keep in mind that flat is better than nested. It is important to strike the right balance.
# Without namespaces
def calculate_square_area(side_length):
return side_length * side_length
def calculate_circle_area(radius):
return 3.14 * radius * radius
square_area = calculate_square_area(4)
circle_area = calculate_circle_area(3)
# With namespaces
class Geometry:
@staticmethod
def calculate_square_area(side_length):
return side_length * side_length
@staticmethod
def calculate_circle_area(radius):
return 3.14 * radius * radius
square_area = Geometry.calculate_square_area(4)
circle_area = Geometry.calculate_circle_area(3)
By using namespaces, we group related functions, classes, or variables under a common namespace, minimizing the chances of naming collisions and enhancing code organization.
Conclusion
As a Python beginner, understanding and embracing the Zen of Python will set you on the path to becoming a proficient developer. Write code that is beautiful, explicit, and simple while prioritizing readability and adhering to Pythonic conventions. With practice and dedication, you’ll soon find yourself crafting elegant and efficient solutions to real-world problems. The Zen of Python will be your guiding light, empowering you to write code that not only works but also inspires and delights.
Happy coding, and welcome to the Python community! As you embark on your Python journey, remember to seek inspiration from the Zen of Python. Let its principles guide you in creating code that not only functions as intended but also reflects the artistry and elegance of Pythonic programming. The adventure awaits — embrace the Zen and unlock the true potential of Python!
Published at DZone with permission of Sushant Mimani. See the original article here.
Opinions expressed by DZone contributors are their own.
Comments