Easy Mapping JSON to Java Objects Using Jackson
Mapping JSON to Java objects just got a lot easier with Jackson.
Join the DZone community and get the full member experience.
Join For Free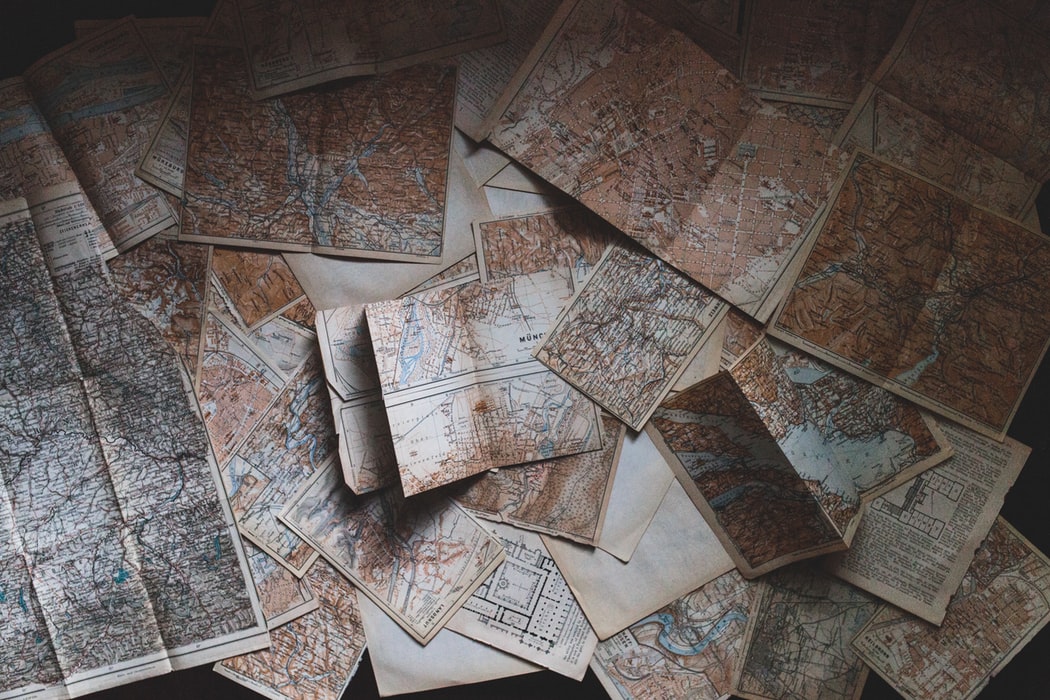
Introduction
Jackson is a very popular and efficient Java-based library used to map JSON into Java objects, and vice versa. In this article, I will demonstrate how easy it is to use Jackson for retrieving data in the JSON response received from REST API and save it to a CSV file.
I will be retrieving the financial data from Elektron Data Platform (EDP), which provides access to Refinitiv various sets of data and will be using historical pricing for the sample.
You may also like: Processing JSON With Jackson
Pre-Requisite
1. EDP access credentials (username, password, and clientId), which can retrieve historical pricing events data. You can contact Refinitiv representatives to acquire username and password and use that to generate clientId at App key Generator.
2. The EDPToken
helper class is used to obtain an access token, which is required before any data requests. You can simply follow this article: Easy Obtaining Token from EDP Gateway in Java.
How it Works
The overview of the process is the following diagram:
Firstly, you need to have a Java class (the top gray box in the diagram), which matches the format of the JSON data response from EDP. The steps to create the Java class are described in Creating Java Class which matches JSON Response from EDP section below.
Then, you can use Jackson ObjectMapper
to read the Java class and JSON response received from the REST API to create a Java object representing the parsed JSON. Then, you can call methods of the Java object to get any values of parsed JSON.
In my application, I will get the values of data key, name, and type in headers key by calling the methods of HistoricalPricingEvent
object. The snipped source code is shown below:
//Jackson ObjectMapper reads HistoricalPricingEvents class and historical pricing events JSON response
//to create an object representing the parsed JSON.
HistoricalPricingEvent eventsData = new ObjectMapper().readerFor( HistoricalPricingEvent.class).readValue(jsonResp);
//get the values of data key in the object
List<List<String>> data = (List<List<String>>)eventsData.getData();
//Get the values of headers key in the object
List<Header> headers= eventsData.getHeaders();
//Get the values of name and type key in headers
Iterator<Header> hiterator = headers.iterator();
while(hiterator.hasNext()) {
Header aheader = (Header)hiterator.next();
String name = aheader.getName();
String type = aheader.getType();
}
The HistoricalPricingEvent
class can be generated by http://www.jsonschema2pojo.org/.
The complete application source code, including theHistoricalPricingEvent
class, can be found on GitHub.
Creating a Java Class to Match the JSON Response From EDP
Instead of writing the full Java class manually, I will use the http://www.jsonschema2pojo.org/ page, which can generate a Java class with Jackson annotation from JSON and JSON schema. Please follow these steps to create a Java class from the JSON schema of historical pricing events data JSON response.
1. Go to API Playground and find the /data/historical-pricing/ service. Then, select the /views/events/{universe} path.
2. Select the Swagger menu to download the JSON schema file.
3. Open the schema file. Under /views/events/{universe}, find the successful response (200 HTTP status code) that contains data. The response refers to the value in /definitions/historicalPricingData path.
Copy the value in the /definitions/historicalPricingData path:
Paste the value in the text box on http://www.jsonschema2pojo.org/
4. Look into the value in the text box, find $ref
, which refers to /definitions/status path:
Copy the value in the /definitions/status path that $ref
refers to:
Replace $ref
line with the copied value:
The complete JSON schema in the text box (the step 3–4) is dataResponseJson.zip.
5. Set Package and Class name e.g. com.java.response and HistoricalPricingEvent
respectively. At Source type, select JSON Schema. The Class name is the name of the Java class that matches the format of JSON schema.
6. Click Zip button. Then, the Java classes are generated and compressed in the zip file. Finally, the zip file name is shown:
Click at the file name to download.
When you uncompress the zip file, you will see the Java class file (HistoricalPricingEvent
) and the related classes as below:
Compile and Run Application
Before you can compile and run the application, you have to set the CLASSPATH
to point to Apache Http Components, JSON in java, Jackson Core, Jackson Annotations, and Jackson Databind like an example on Windows machine below:
xxxxxxxxxx
set CLASSPATH=C:\thirdPartyJars\httpcomponents-client-4.5.9\lib\commons-codec-1.11.jar;C:\thirdPartyJars\httpcomponents-client-4.5.9\lib\commons-logging-1.2.jar;C:\thirdPartyJars\httpcomponents-client-4.5.9\lib\httpclient-4.5.9.jar;C:\thirdPartyJars\httpcomponents-client-4.5.9\lib\httpcore-4.4.11.jar;C:\thirdPartyJars\JsonInJava\json-20180813.jar;C:\thirdPartyJars\commons-cli-1.4\commons-cli-1.4.jar;C:\thirdPartyJars\Jackson\jackson-annotations-2.9.9.jar;C:\thirdPartyJars\Jackson\jackson-core-2.9.9.jar;C:\thirdPartyJars\Jackson\jackson-databind-2.9.9.jar
The Java command line to request the latest five quote events of item name “THB=,” which is Thai Baht is below:
xxxxxxxxxx
java -classpath %CLASSPATH%;. com.java.HistoricalPricingEventsRequestor -user <EDP username> -clientId <clientId> -rics THB= -events quote -count 5
The console will display the following once successful:
You can now check the historical pricing events data in a CSV file like an example below:
Summary
This is just a sample to explain how to use Jackson to map JSON to Java objects. Then, you can easily manage the Java objects in various ways. It also suggests http://www.jsonschema2pojo.org/, which can generate the Java class from the JSON response schema. Jackson requires this Java class for parsing a JSON response into an object of this Java class. This can be more automated especially with the schema file access and update, which can be found in APIDocs for other APIs.
Further Reading
Opinions expressed by DZone contributors are their own.
Comments