Configuration As A Service: Spring Cloud Config – Using Kotlin
In this article, we will demonstrate the Spring cloud config server using Kotlin. Spring Boot provided a much-needed spark to the Spring projects.
Join the DZone community and get the full member experience.
Join For FreeDeveloping a microservice architecture with Java and Spring Boot is quite popular these days. In microservice architecture we hundreds of services and managing services for each service and for each profile which is a quite tedious task. In this article, we will demonstrate the Spring cloud config server using Kotlin.
Spring Boot provided a much-needed spark to the Spring projects.
Spring cloud-config provides a server and client-side support for externalizedconfiguration in a distributed system. With the Config Server, you have a central place to manage external properties for applications across all environments.
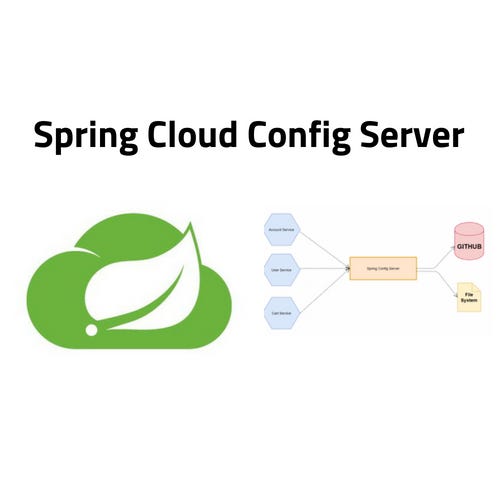
From the above diagram, you can easily predict that in distributed systems managing configuration as a central service is a bit tedious task and spring cloud config provide client, server architecture mechanism to manage the configuration easily.
Let us go to the https://start.spring.io/
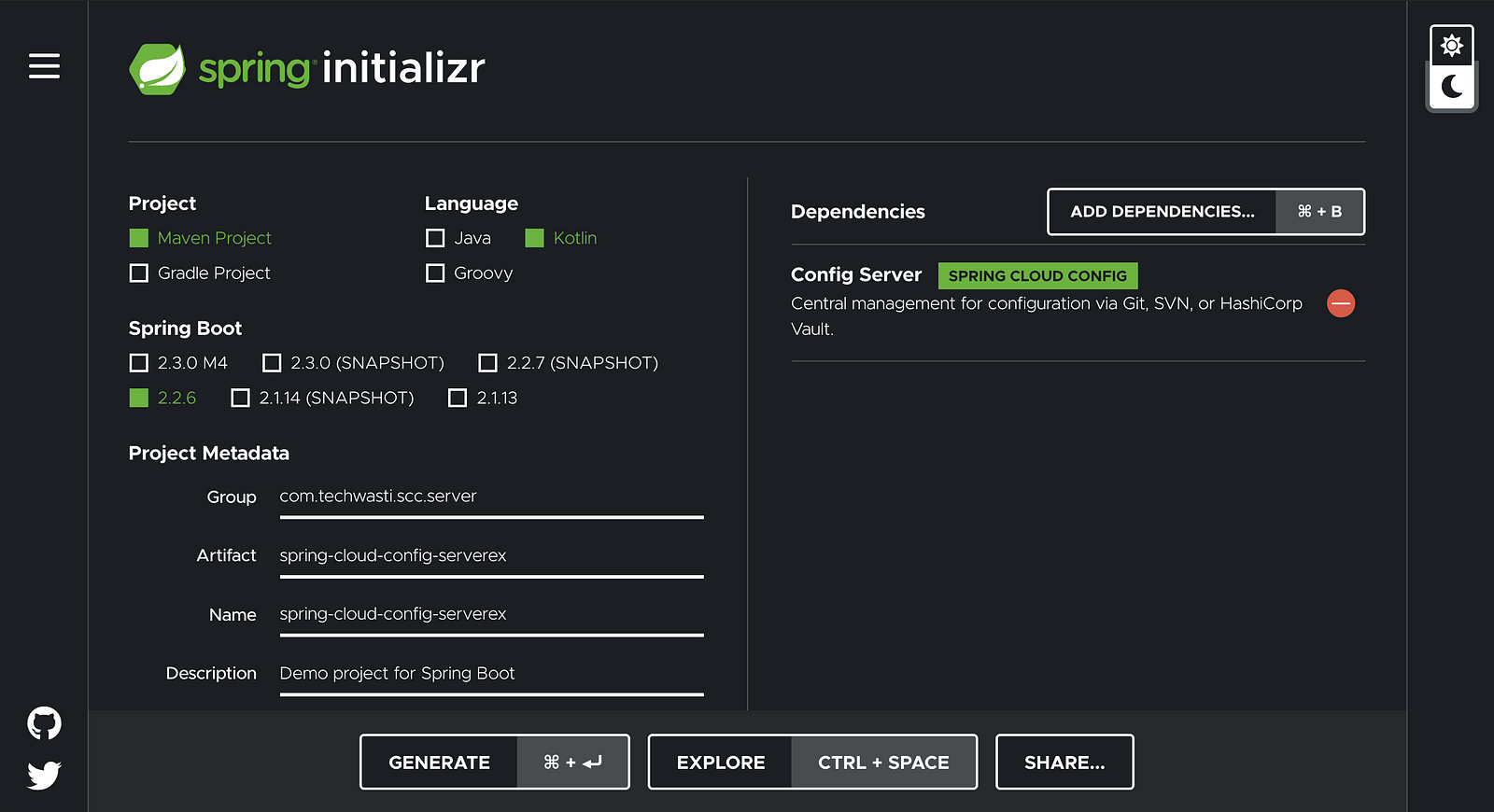
When we do any changes in any service we have to restart the services to apply the changes.
Let us create one git repo to manage our configuration and to achieve this we are creating one git repo.
So we will create “springbootclient” as one small spring boot microservice to take the username and that will read username from spring cloud config central configuration server system i.e git here.
We have created three different properties files for each of our different environments.
- springbootclient.properties
- springbootclient-dev.properties
- springbootclient-prod.properties
https://github.com/maheshwarLigade/cloud-common-config-server
Here is our spring cloud config properties are available, you can clone or use directly this repository too.
Now as we have created spring config server application using spring starter let us download and import that project in your favorite IDE or editor. git repo here we used to store our configuration and spring cloud config server application is to serve those properties to the client.
Basically git is datastore, spring cloud config server is server application and there are multiple microservices are the clients which needs configurations.
Now our git as datastore is ready. In this repository, we have created one sample client application and the name of that app is springbootclient. In the future microservice article we will utilize the same spring cloud config as a configuration server.
Let us go and check the code base for the client app.
This is the sample application.properties file:
server.port=8888
logging.level.org.springframework.cloud.config=DEBUG
spring.cloud.config.server.git.uri=https://github.com/maheshwarLigade/cloud-common-config-server.git
spring.cloud.config.server.git.clone-on-start=true
spring.cloud.config.server.git.searchPaths=springbootclient
Sample Code for SpringCloudConfigServerexApplication.kt
xxxxxxxxxx
import org.springframework.boot.autoconfigure.SpringBootApplication
import org.springframework.boot.runApplication
import org.springframework.cloud.config.server.EnableConfigServer
class SpringCloudConfigServerexApplication
fun main(args: Array<String>) {
runApplication<SpringCloudConfigServerexApplication>(*args)
}
Now run and up the spring cloud-config server and check the below URL:
http://localhost:8888/springbootclient/dev/master
Spring Boot Client App
Let us create one small microservice which will read configuration from the spring cloud config server and serve that property value over the REST endpoint.
Go to the https://start.spring.io/ and create spring boot client microservice using kotlin.
Sample POM.xml dependencies.
xxxxxxxxxx
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.module</groupId>
<artifactId>jackson-module-kotlin</artifactId>
</dependency>
<dependency>
<groupId>org.jetbrains.kotlin</groupId>
<artifactId>kotlin-reflect</artifactId>
</dependency>
<dependency>
<groupId>org.jetbrains.kotlin</groupId>
<artifactId>kotlin-stdlib-jdk8</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-config</artifactId>
</dependency>
Now check the SpringCloudClientAppApplication.kt code
xxxxxxxxxx
import org.springframework.boot.autoconfigure.SpringBootApplication
import org.springframework.boot.runApplication
class SpringCloudClientAppApplication
fun main(args: Array<String>) {
runApplication<SpringCloudClientAppApplication>(*args)
}
Now create one sample REST controller which is serving REST request. We want to check ” /whoami” this endpoint is returning which is the user based on active profile dev, prod, etc.
UserController.kt
xxxxxxxxxx
import org.springframework.beans.factory.annotation.Value
import org.springframework.web.bind.annotation.GetMapping
import org.springframework.web.bind.annotation.RestController
class UserController {
"\${app.adminusername}") (
var username="Test"
//get request serving
"/whoami") (
fun whoami() = "I am a "+ username
}
Create a bootstrap.properties file where we will specify the spring cloud config server details, which is a git branch and what is active profile dev, local, prod, etc.
xxxxxxxxxx
spring.application.name=springbootclient
spring.profiles.active=dev
spring.cloud.config.uri=http://localhost:8888
spring.cloud.config.fail-fast=true
spring.cloud.config.label=master
All properties are self exclamatory, what is the use of which one.
Once you hit this URL http://localhost:9080/whoami
Output: I am a DevUser
Github source link:
Config Server: https://github.com/maheshwarLigade/cloud-common-config-server
Codebase: https://github.com/maheshwarLigade/spring-cloud-config-kotlin-ex
Opinions expressed by DZone contributors are their own.
Comments